Introduction
Object-oriented programming (OOP) is a programming model centered on the concept of 'objects,' which can include both data and code: field-like data (commonly called attributes or properties) and code as processes (often known as methods).
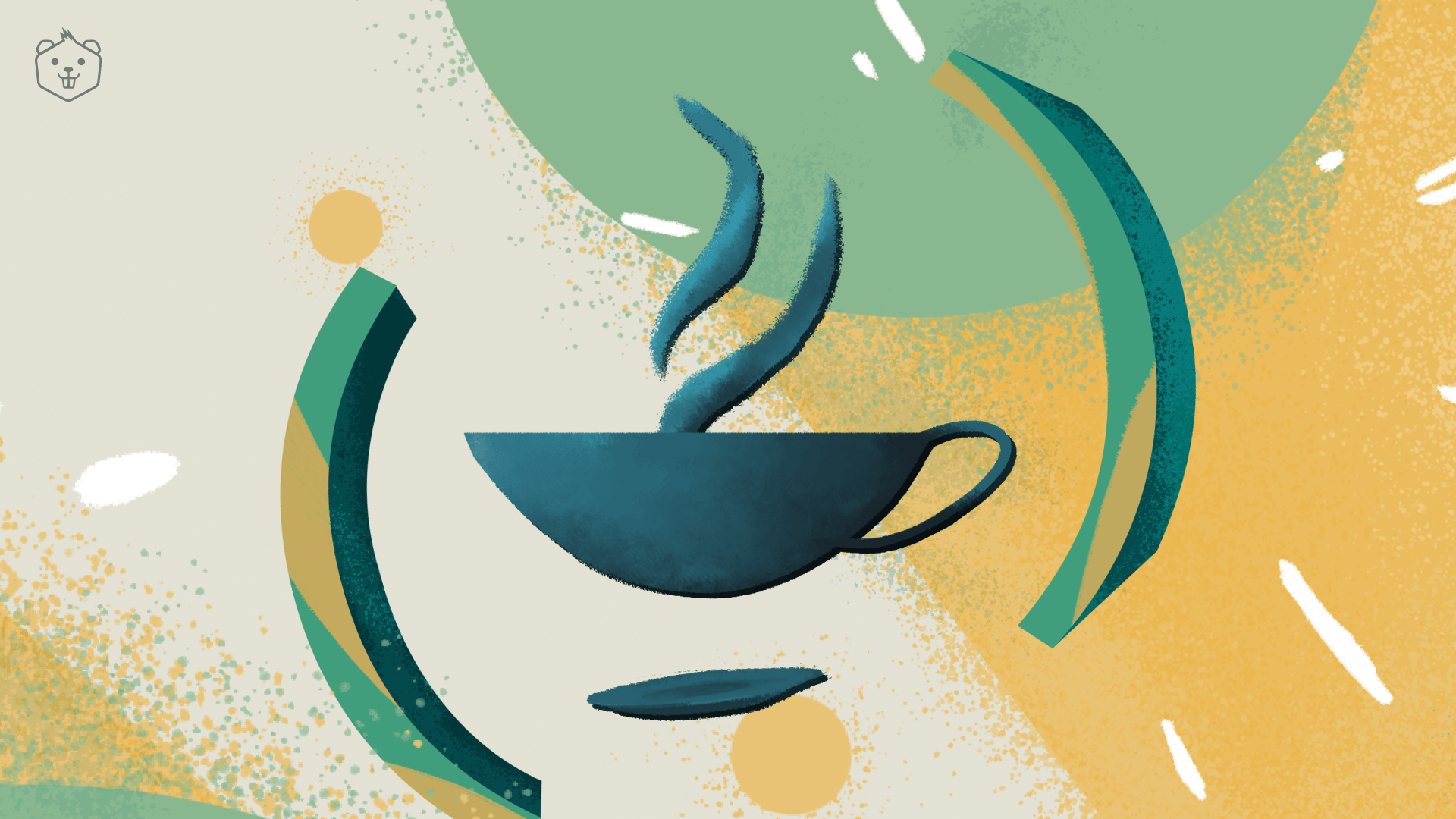
One characteristic of objects is that the processes of an object itself can access and often edit its data fields (objects have a notion of this or self). Computer programs in OOP are meant to create objects that interact with each other. There are a lot of OOP languages but class-based OOP languages are most prevalent, wherein objects are class instances that also specify their attributes and behaviour.
Also check out: Crio Bytes to master OOPs foundations with hands-on activities
Understanding object-oriented programming in C++
The primary objective of C++ programming is to introduce an object-oriented approach to C programming.
Object-oriented programming is a paradigm that offers several concepts such as inheritance, data binding, polymorphism, encapsulation, and many more. We'll be exploring more about polymorphism in this blog.
There are a few fundamental concepts that form the groundwork of object-oriented programming in C++. They are listed below:
Class
When you create a class, you're essentially creating a blueprint for an item. This doesn't specify any data, but it does define what the class name implies, that is, what a class object will be made up of and what actions can be done on it.
To create a class, we use the predefined 'class' keyword in C++ as shown below:
Notice that we have defined a new class 'Student' which contains a few attributes including name, age, and roll number.
Object
A Class instance is an Object. When a class is declared, no memory is allocated; nevertheless, memory is allocated when it is instantiated (i.e. when an object is formed). Here's a simple example in C++:
Output
In the above example, we defined a new object 's1' which belongs to the class 'Student'. After initializing the data members of the class, we print out the values to the console.
Encapsulation
Encapsulation is the process of embedding data and the functions that deal with it in the same space. It's not always apparent which functions work on which variables when dealing with procedural languages, but object-oriented programming gives you a framework for putting data and relevant functions together in the same object.
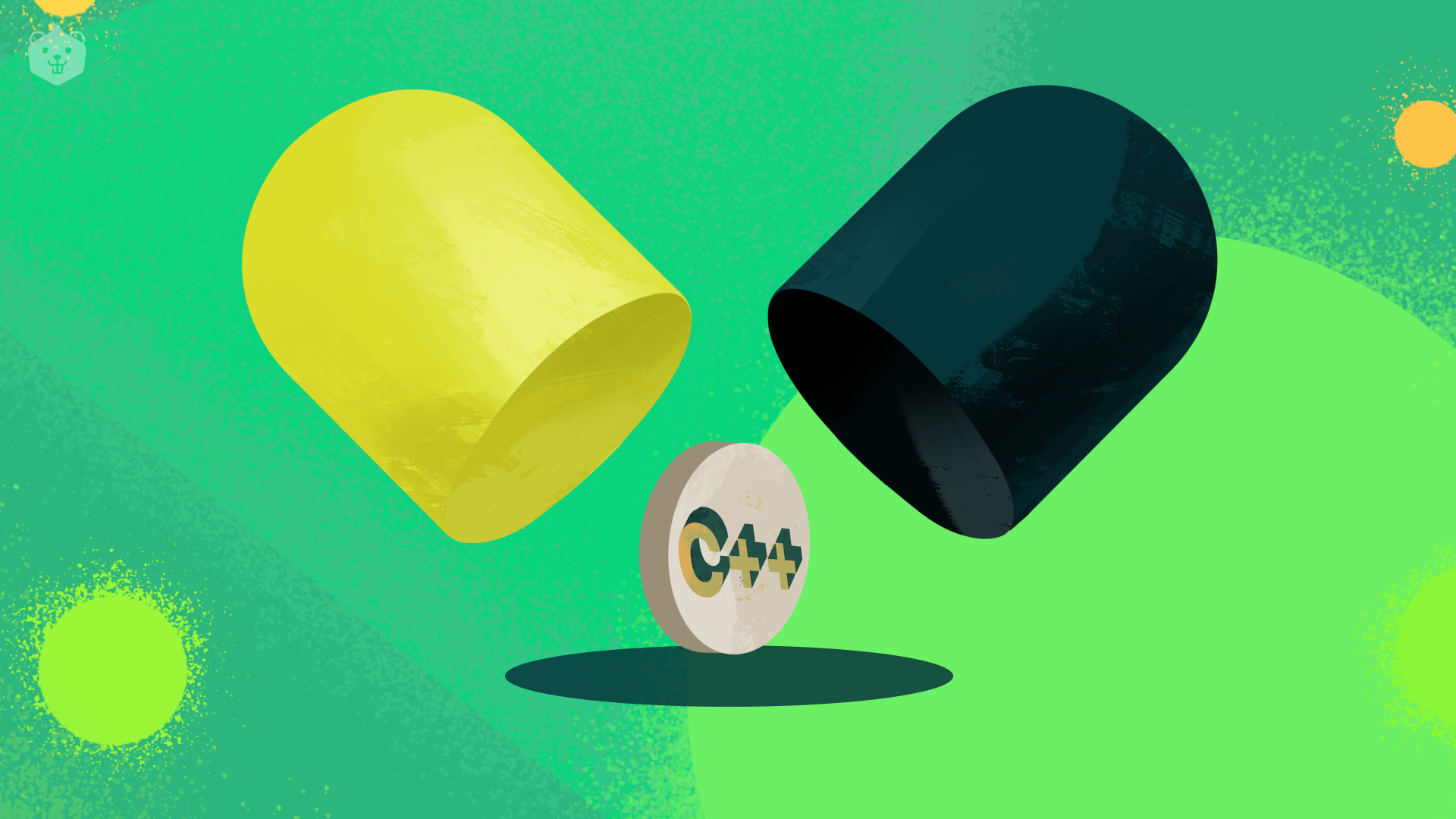
Abstraction
Data abstraction is defined as exposing only important information to the outer world while masking background details, i.e., representing the required information in a program without showing the precise details.
A database system, for example, conceals certain aspects of data storage, creation, and maintenance. Similarly, C++ classes expose many methods to the outside world without disclosing any internal information about those functions or data.
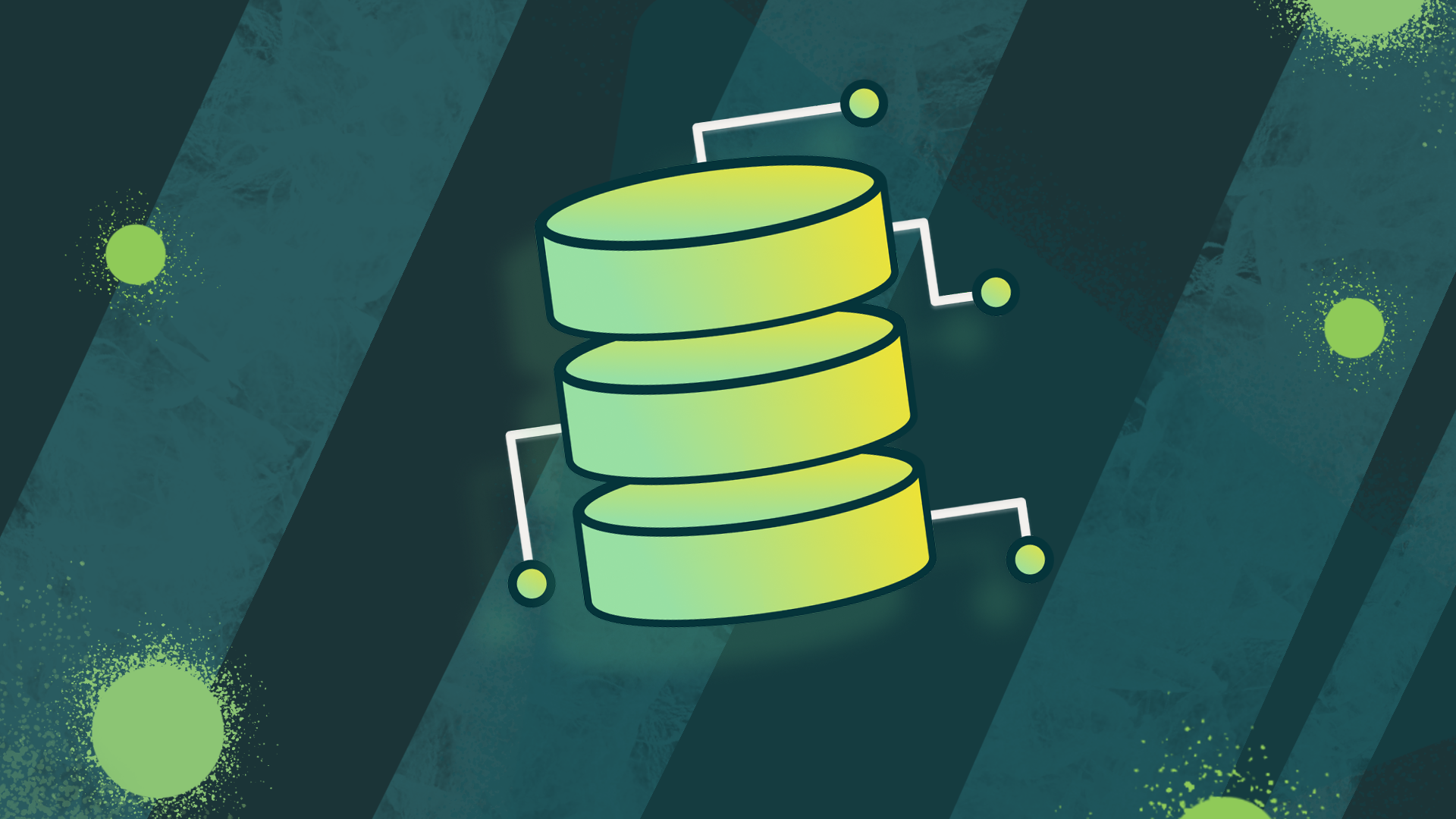
Inheritance
One of the most significant features of Object-Oriented Programming is inheritance. Inheritance refers to a class's capacity to derive features and traits from another class.
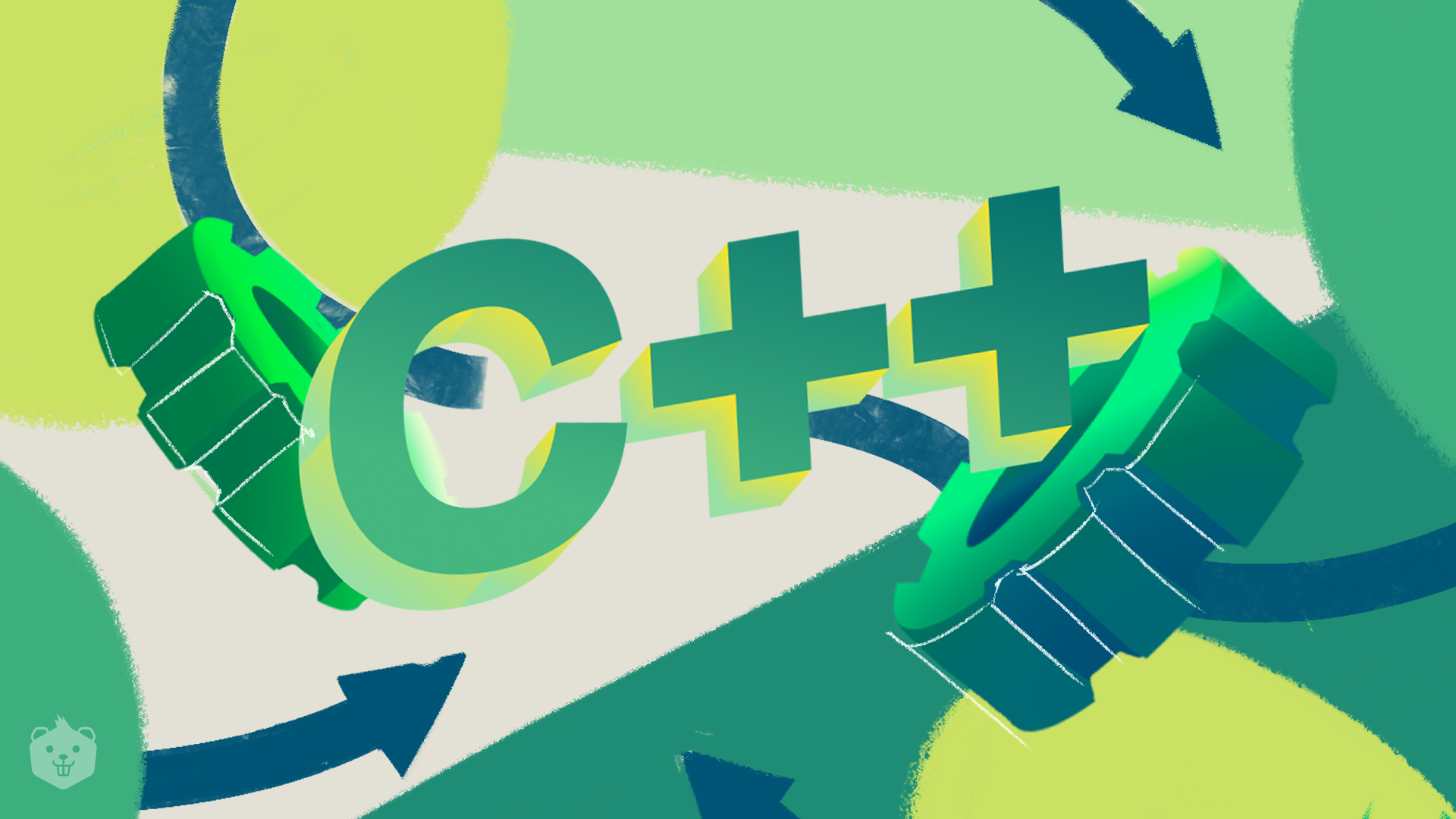
Polymorphism
Polymorphism is the flexibility to employ an operator or function in several ways, or to give the operators or functions alternative meanings or functions.
Polymorphism refers to a single function or operator that can function in a variety of ways depending on the context.
Exploring Polymorphism in C++
In simple terms, polymorphism is the ability of a message or object to be shown in multiple forms. That is, in different scenarios, the same entity (function or operator) behaves differently. Here's an example to demonstrate this concept,
Output
In the above C++ program, we can observe that the '+' operator exhibits different behaviour under different circumstances. To clarify, the '+' operator performs addition on the two integer operands but concatenates the values of the two string operands.
Implementation of Polymorphism in C++
Here's a flowchart to depict the different types of polymorphism in C++
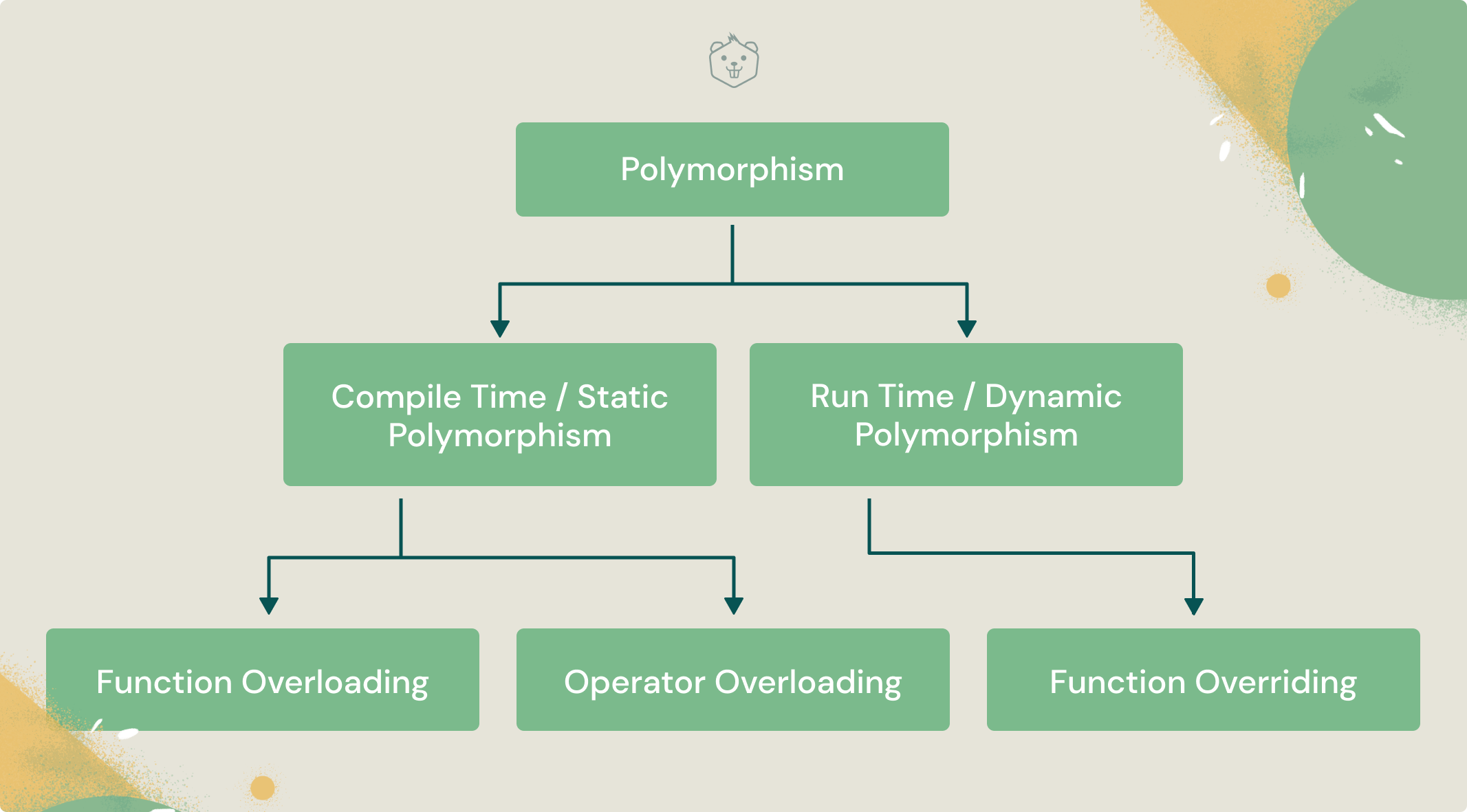
Function Overloading (Compile Time Polymorphism)
Definition
Function overloading, also known as method overloading, is the ability to construct numerous functions with the same name but distinct implementations in some programming languages. Calls to an overloaded function will execute a specific implementation of that function tailored to the context of the call, allowing a single function call to accomplish multiple tasks depending on the situation.
Code
Output
Explanation
In the above C++ program, there are three separate functions defined with the same name but are easily distinguishable by their parameters. For the first add() function, the parameters are integers and thus the first function call statement invokes this add() function because we exclusively pass integers as arguments.
Similarly, for the second add() function, the parameters are defined with 'double' datatype and thus the second function call statement invokes this add() function because we exclusively pass floating-point numbers as arguments.
Thus we successfully implement the concept of polymorphism through function overloading in C++.
Operator Overloading (Compile Time Polymorphism)
Definition
Operator overloading, also known as operator ad hoc polymorphism in computer programming, is a type of polymorphism in which various operators have multiple implementations based on their parameters. A programming language, a programmer, or both can define operator overloading.
Code
Output
Try it yourself
Q1. What will be the output of the following C++ program?
Q2. Predict the output of the following C++ program given below.
Function Overriding (Run Time Polymorphism)
Definition
In C++, function overriding occurs when a derived class defines the same function as its base class. It's used to achieve polymorphism at runtime. It allows you to create a custom implementation of a function that is already available in the base class.
Code
Output
Explanation
As you can see above, the display() function is defined in both the Parent and Child classes. Now, when the display() function is invoked from the Child object 'obj', the display() function inside the derived function is executed by overriding the display() function inside the Parent class. That's exactly what function overriding is all about.
Try it yourself
Q1. Destructors can be overloaded in C++.
- True
- False
Q2. What is the maximum limit to the number of classes in a single C++ program?
- 1
- 99
- 9999
- Unlimited
Real-World Examples of Polymorphism
- Consider your smartphone. You can save contacts in it, right? Yes. Now it's quite common for an individual to have more than one phone number for himself/herself in the current generation. The fact that you can save more than one phone number under the same name in your mobile device represents polymorphism wherein the given name (your friend's name for instance) has multiple definitions (here, phone numbers) associated with it.
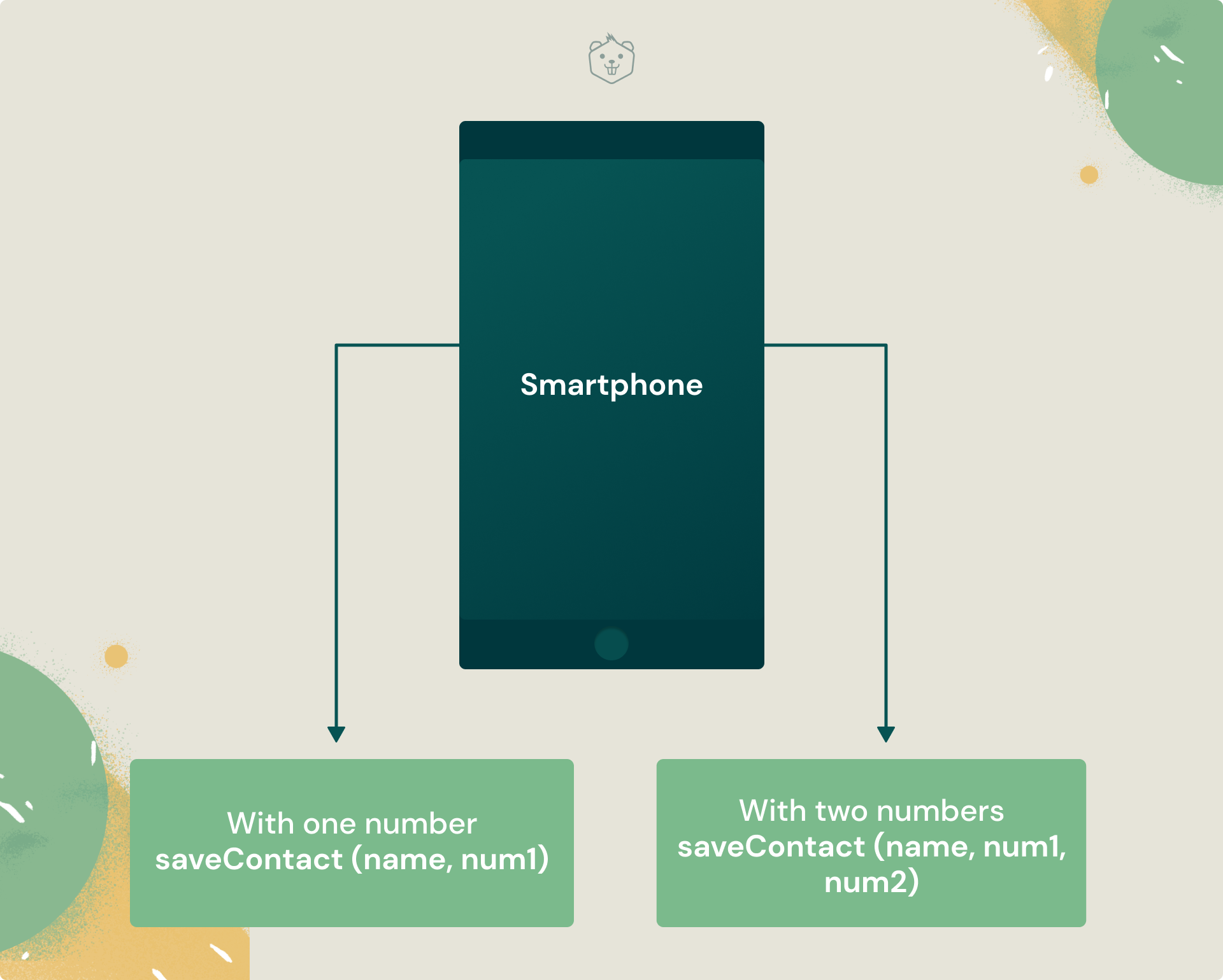
- Another simple example can be cited from the English grammar wherein words have multiple definitions/meanings associated with them. Here's a simple example. The word 'bark' has two distinct interpretations under different circumstances. Look below:
1. bark: Outermost woody layer of trees and stems in rooted plants (bark of a tree)
2. bark: Sharp cry of a dog or a seal (dog's bark)
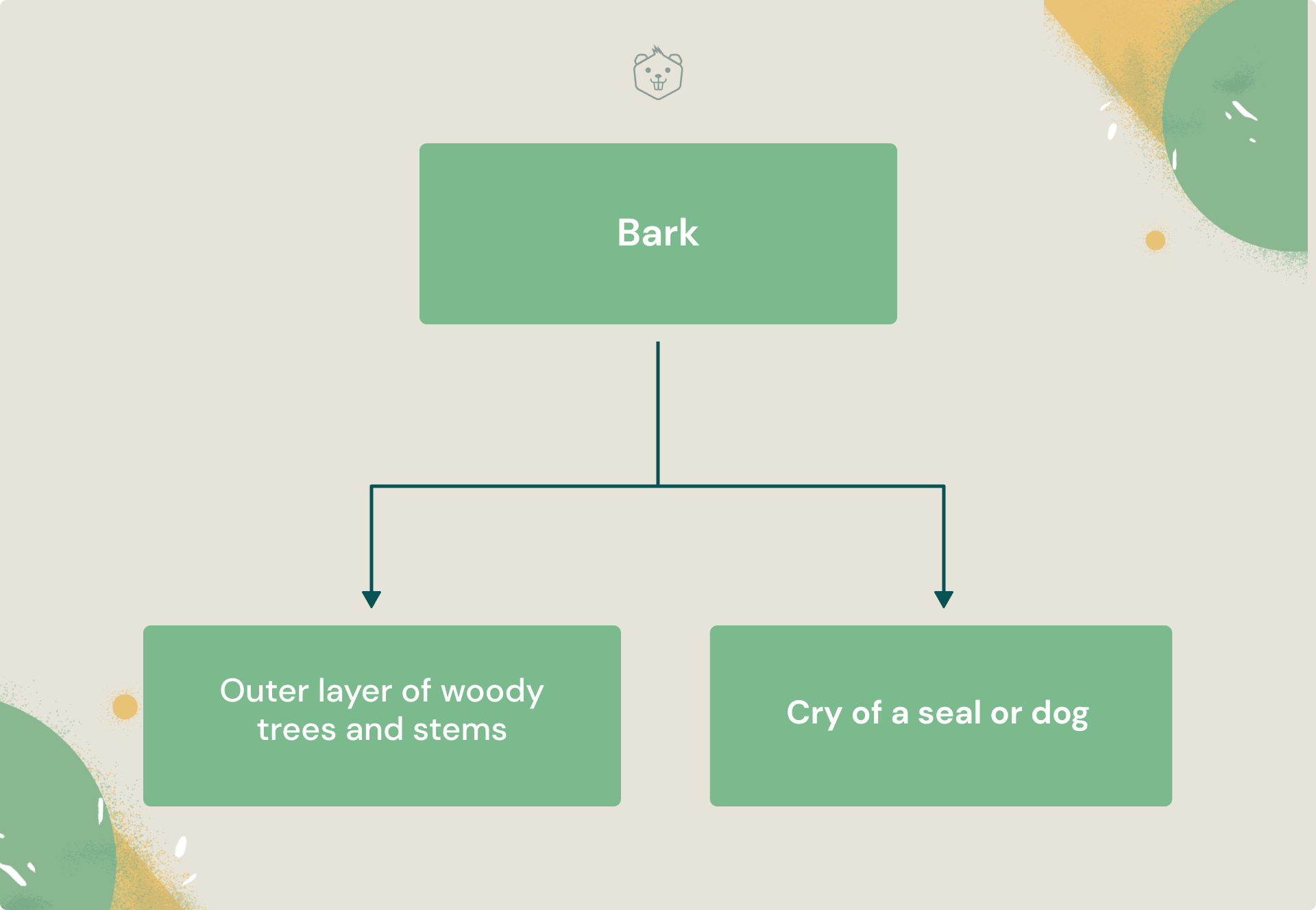
Advantages of polymorphism
Polymorphism is a powerful object-oriented programming concept and has several benefits in the real world. Some of the most impactful benefits are listed below:
- It implements code reusability, i.e. pre-written, pre-tested classes can be reused as required. This cuts down on a lot of time.
- Single variable naming can be used for multiple data types in C++
- Cuts down on coupling between different functionalities in C++
- Builders that allow different ways of initializing class objects can use method overloading as well. It assists you in identifying a number of builders for dealing with distinct types of initializations.
Final Thoughts
By now you will have already noticed how powerful polymorphism is in the real world. Programmers and developers leverage this all the time to cut down on their development time and build better products in the long run. There's so much more to polymorphism but this blog should be good enough to build a strong foundation for yourself in C++.
Also read:
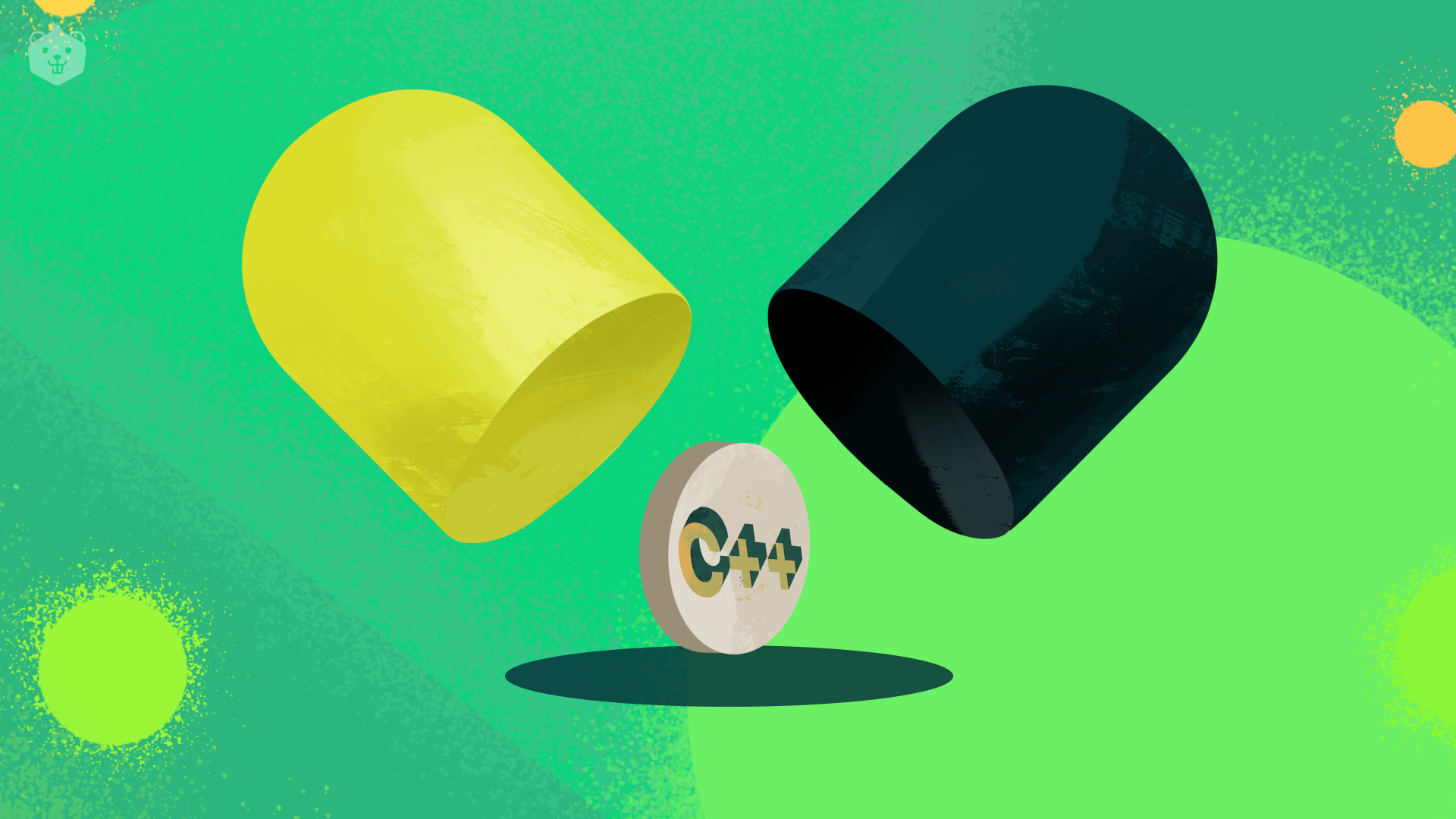
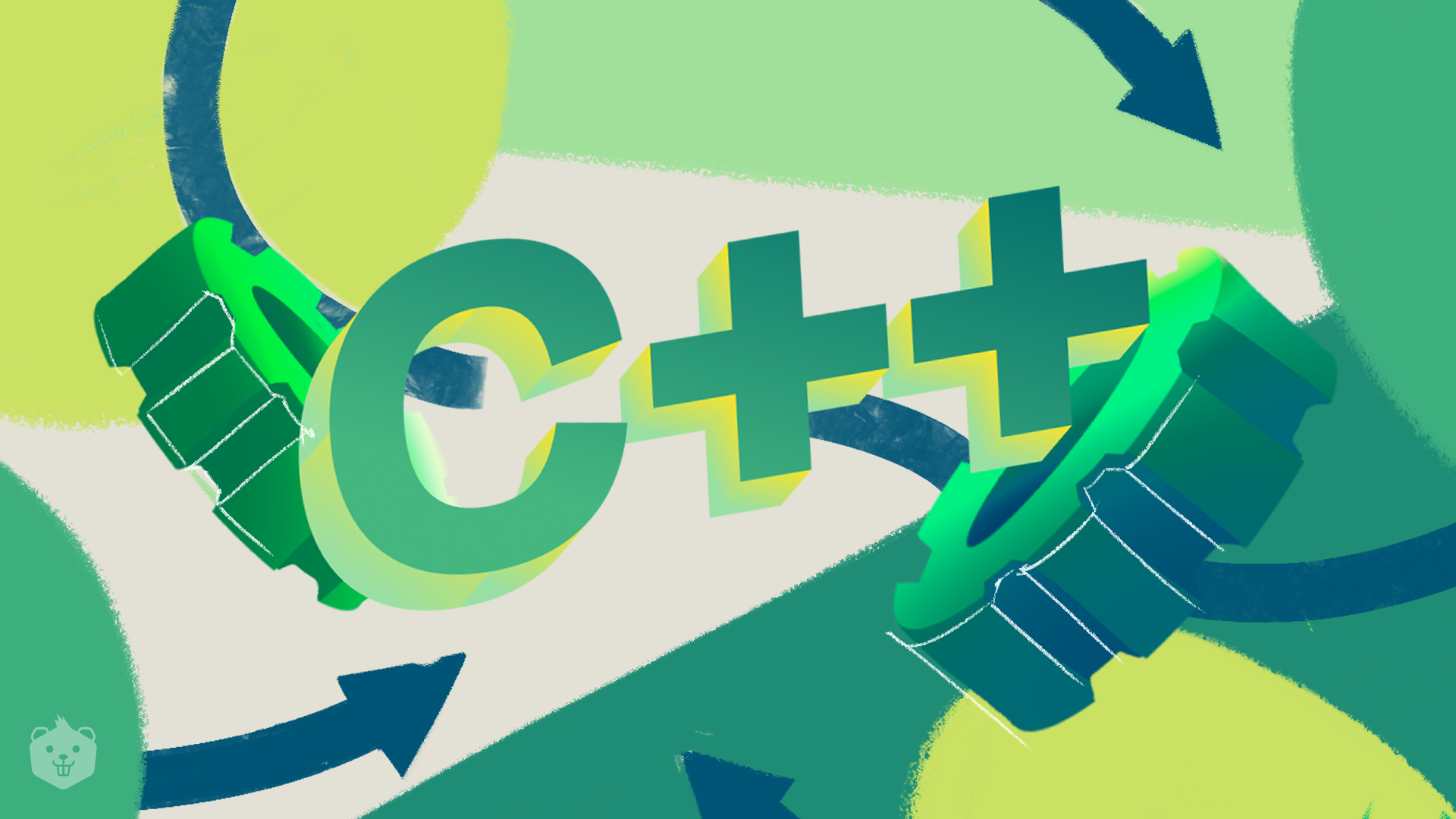
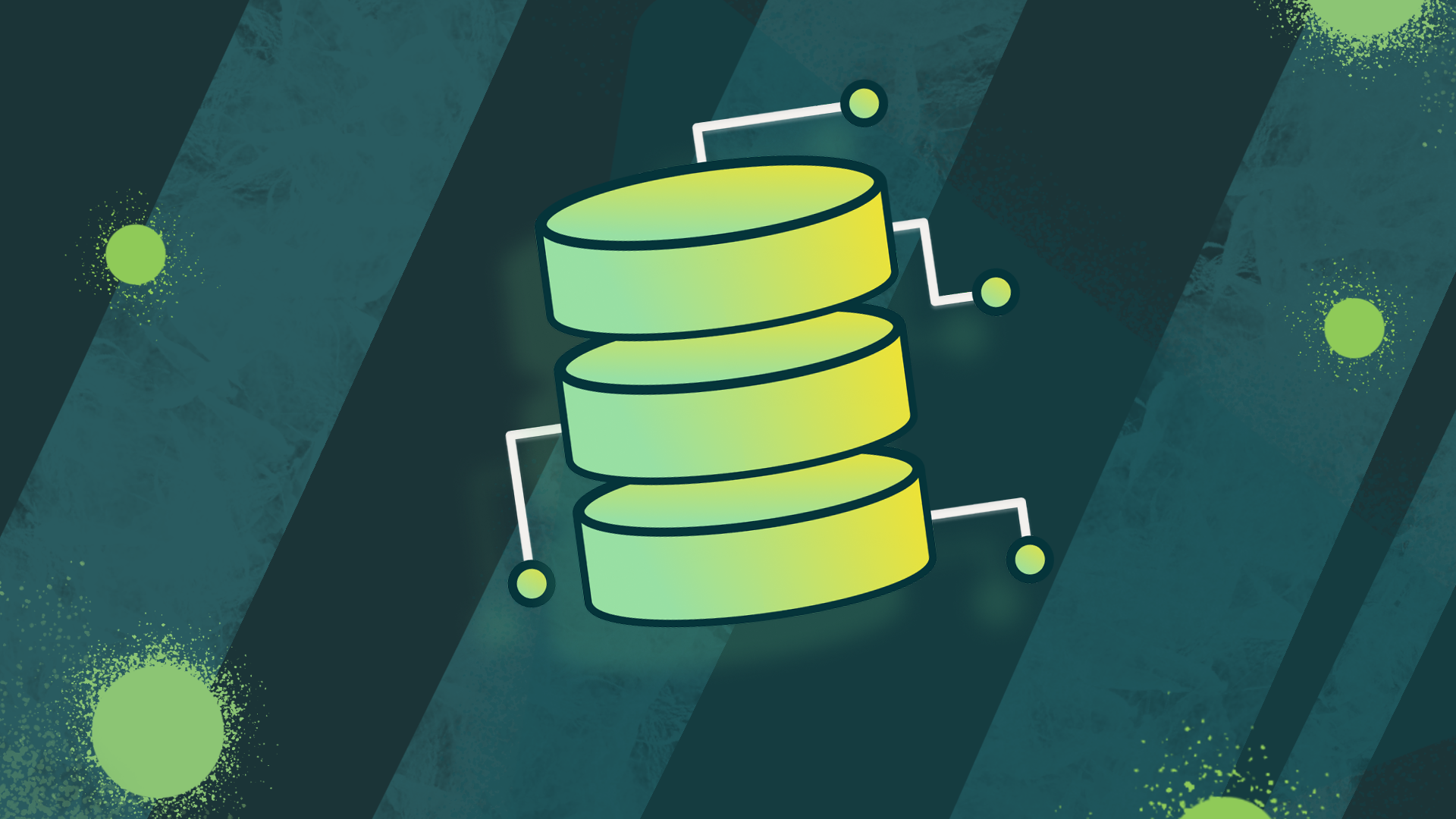
Did we miss out on something? Let us know in the comments below.
Enjoyed this article? Leave a like below or share it with your friends via your social media handles.