Computers can only comprehend binary numbers i.e. 0 and 1. Isn't that correct?
Humans, on the other hand, are mainly concerned with Strings.
Strings are widely used in almost all application codes. As a result, robust support for string composition and manipulation is necessary for a programming language to be effective both in terms of storage and time.
And as the industry's favorite, Java does this very well.
Before we find out how let’s discuss -- What exactly is a String?
A string can be thought of as a stream of characters that are supported by the programming language you are using and represents textual data.
Exploring String Class in Java
In Java, Strings are an instance of the String class and Double quotes “ ” are used to represent Strings in Java.
Examples of Strings in Java
- “Project-based Learning”
- “Java NOob 2 Expert!!”
- “abc”
How do we create strings in Java?
A String object can be created in one of two ways:
- String Literal - Double quotes are used to construct string literals.
- Using new: Using the keyword "new," a Java String is generated.
Let's look at each.
String Literal - Double quotes are used to construct string literals.
Example: String greetString = “Welcome”;
This creates a String object in Java String Pool also referred to as String Constant Pool (SCP) which is a special place for all strings in Heap Memory.
Why is this special area in heap(SCP) required? Is it only for Strings?
Yes it is only for strings mainly for 2 reasons
- Most Used Objects
- Immutability of Strings
By storing only one copy of each literal String in the pool, JVM will optimize the amount of memory allocated for strings. When we declare a String variable and assign it a value, the JVM scans the pool for a String of the same value. If it is located, the compiler would automatically return a reference to its memory address rather than allocating additional memory.
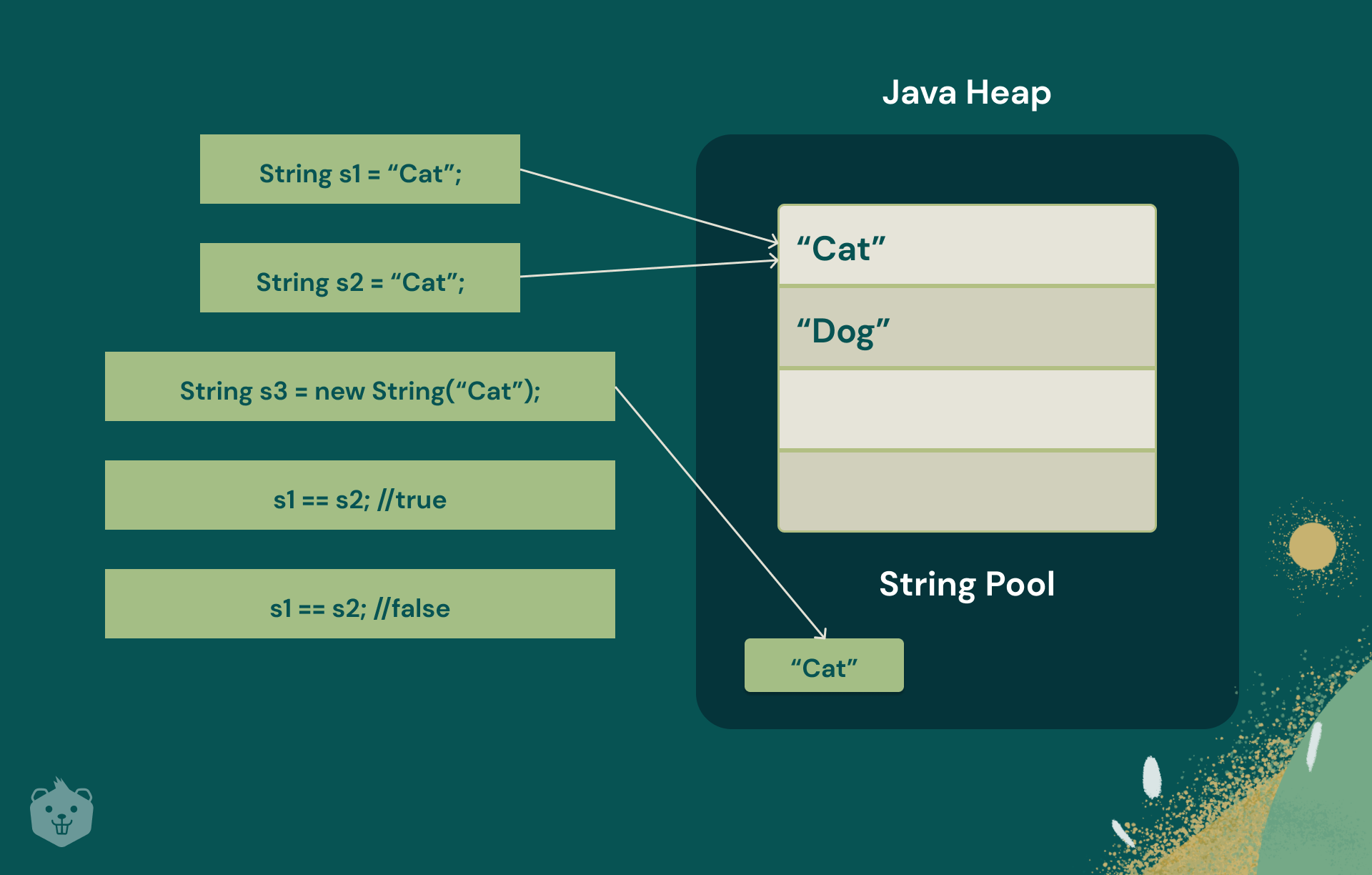
2. Using new: Using the keyword "new," a Java String is generated.
Example: String greetString = new String(“Welcome”)
This declaration creates a string object in heap memory and checks to see if it is in the string pool. If the string “Welcome” is not in the string pool, it will add it; otherwise, it will skip it and return a reference variable, which refers to the newly created heap object.
To read more about SCP glance through - https://www.baeldung.com/java-string-constant-pool-heap-stack
How and why is it useful to use String functions in Java programming?
The String class contains over 60 methods that serve as basic utility methods to make the life of a developer dealing with strings smoother.
Note about Immutability Of Strings
Since the String class is immutable, all string objects generated with literals or new operators cannot be altered or updated. At the time of creation, the value of the immutable class object is set. Immutability of strings has numerous advantages, including improved performance due to the String Pool model, security, and thread safety.
Quick Exercise to demonstrate this concept is given below
You must use different classes, such as StringBuilder that are mutable counterparts of String if you wish to move the String to different methods for modification.
What if you plan to write your own implementation of existing methods?
That's a perfect way to practice and improve your Java skills, but it's definitely not a good idea to use it in production.
The primary reason for this is that you might easily introduce bugs into your code. The Java Community is vast, and these implementations have been thoroughly reviewed and tested in stringent environments and are well known. Instead of reinventing the wheel, you should concentrate on your business logic. Another reason includes inbuilt documentation support, enhances the readability of code, and continued support from the community.
For example, to find out the number of characters contained in the string object, length() method can be used instead of iterating over all characters and increasing the count one at a time.
Let’s dive deep into the most commonly used Java string methods and understand their working.
1. indexOf()
Used to find characters and substrings in a string. It returns the index of the first occurrence from the left of the passed string within this string; moreover, if we provide the fromIndex, it starts searching from that index.
Syntax of indexOf()
Parameter(s)
- str: Substring to be searched for
- fromIndex: Search begins from this index
Return Value
Index of the first occurrence of the specified substring, 0 if an empty string is passed, or -1 if there is no such occurrence.
Overloaded Variants
Real world application of indexOf()
Ever wondered how your favorite search feature in Code Editors works?
indexOf() can be used to implement this feature. How?
The idea is to iterate over all of the lines and invoke indexOf() with the target string, and if there are multiple occurrences of the target string in the same line, start from where the last found substring ends.
Try it yourself
Write a function that uses indexOf() to find if a given character is a vowel or not.
Input - any character; Output - true if a given character is vowel else false
Don't forget to share your unique approach in the comments below.
2. toCharArray()
Used to form a new character array from this string. The newly allocated array's contents are initialized to contain the characters represented by this string, and its length is the length of this string.
Syntax of toCharArray()
Parameter(s)
None
Return Value
char[] array is created in memory with content as characters of the string and its reference is returned
Real world application of toCharArray()
As we have discussed above, string objects are immutable.
During heavy string manipulation operations, many new string objects are made and this can be memory inefficient. A good idea is to convert it to char Array first, then perform manipulation operations and then convert it back to a string object.
Let’s take an example to see if a given string is palindrome or not.
Try it yourself
Find all the indices of uppercase letters present in the string and convert them to their ASCII value.
Example - Input - asBcDeE Output - [2,4,6] modified string - as66c68e69
Let us know your most optimal approach in the comments below
3. charAt()
The character at the designated index (follows 0 based indexing) is extracted from the string. It's useful for checking a certain index without looping over the whole string.
Syntax of charAt()
Parameter(s)
- Index: index of character in string to be retrieved
Return Value
char value from String at index passed as argument
- Throws
IndexOutOfBoundsException - if the argument provided is negative or greater than the length of String.
Real world application of charAt()
Counting the frequency of a particular character - charAt() can be used to iterate the string and count frequency of a character.
Try it yourself
Print the characters along with their frequency in the order of their occurrence.
Example - Input - “learnByDoing”
Output - [[l, 1], [e,1], [a,1], [r, 1], [n, 2], [B, 1], [y,1], [d,1], [o, 1], [i,1], [g,1]]
Let us know your most optimal approach in the comments below.
4. concat()
The passed string is appended to the end of the specified string. It's a great option for combining/merging strings.
Syntax of concat()
Parameter(s)
str: string to be appended at end of a given string
Return Value
A new String object with a passed string appended at the end of the given string.
Real world application of concat()
You must have filled many forms online and encountered fields like Address Line 1, Address Line 2.
Do you think these are stored as it is in the database?
You might be wrong if you think so. When form data is processed it is combined to form a single field like a full address.
Try it yourself
While entering credit/debit card details you must have encountered multiple fields to fill in entering the card number. How will you combine them and verify with the server that your card number is valid?
Assume that server stores number in filed credit card number in the format 1234-567-1239
Let us know your most optimal approach in the comments below.
5. replace()
Used for replacing characters and substrings in a string.
Syntax of replace()
Parameter(s)
- oldChar: Character that needs to be replaced
- newChar: Character that will replace the oldChar
Return Value
A new String object containing a string created by replacing all instances of oldChar with newChar.
Real world application of replace()
If you are a programmer, you might have encountered a scenario in which you needed to modify the name of all instances of your variable. How would you put this feature into action?
Try it yourself
Given an array of Strings. Remove all the whitespaces present in all the strings that are part of this array.
Example - Input - [[‘hey this is ’], [‘a example of’], [‘replace method ’]];
Output - ‘heythisisaexampleofreplacemethod’
Let us know your most optimal approach in the comments below.
6. substring()
Used to extract a portion of a string from a given string. It creates a new string object without altering the original string.
Syntax of substring()
Parameter(s)
- beginIndex: Search begins from this index
- endIndex: Search end at endindex-1
Return Value
A new String object resulting from the part of the original string sliced out on the basis of begin and endIndex.
- Throws
IndexOutOfBoundsException - if beginIndex is negative or larger than the length of this String object.
Overloaded Variant
Real world application of substring()
We can find out all the substrings of a given string using substring(). Though this is not an optimal approach, it will help you through the learning of the substring method’s working.
Try it yourself
Find out if the given string is palindrome or not by using substring().
Share your solution with fellow readers in the comments section below.
7. split()
Used to separate the specified string depending on the regular expression. The limit statement is used to limit the number of strings returned after separating.
Syntax of split()
Parameter(s)
- regex: Substring to be searched for
- limit: Search begins from this index
Return Value
An array containing each substring of this string is ended by a substring that matches the given expression or by the end of the string. The array's substrings are listed in the order in which they appear in this string.
- Throws
PatternSyntaxException - if the regular expression's syntax is invalid
Overloaded Variants
Real world application of split()
We can count the number of words present in a paragraph by splitting the string by passing space as an argument.
Try it yourself
Given a date string in DD/MM/YYYY format, extract and print the year given in the string.
Example - Input - 26/09/2000 Output - 2000
8. compareTo()
As Java developers, we often need to sort items that are grouped together in a list or in a class, and compareTo() is used to compare two Strings alphabetically.
Syntax of compareTo()
Parameter(s)
- anotherString: String to be compared
Return Value
0 if the argument string is equal to given string;
Value < 0 if the given string is lexicographically less than the string argument;
Value > 0 if the given string is lexicographically greater than the string argument.
Real world application of compareTo()
Comparison-based sorting algorithms are the most used sorting algorithms.
In educational institutes mostly students are arranged based on increasing the order of their firstName. If the firstName of 2 students is the same then decide on the basis of increasing order of their lastName.
Try it yourself
Use compareTo() to find the length of a string.
Hint: Closely examine what compareTo() returns and how this can help us to find the length of the string.
Mention your approach in the comments section.
9. strip()
To eliminate all trailing and leading whitespaces from the specified string.
Syntax of strip()
Parameter(s)
None
Return Value
Returns a string whose value is this string, with all leading and trailing white space removed.
Real world application od strip()
When taking input from your presentation layer, you must ensure that trailing and leading whitespaces do not contaminate the actual string. This ensures the data is consistently stored in the database and processed in the backend.
split() can be used to ensure that our input is not polluted.
Try it yourself
What happens if we don't delete the whitespaces? Does it have an effect on our business operations, or is it just a way to keep things clean? Find out by taking any leading spaces in a string and comparing it to the original string with no leading spaces.
Mention your observations in the comment section below.
10. valueOf()
Used to return string representation of the passed argument. valueOf() has a plethora of overloaded variants that aid in the conversion of almost any primitive form to string.
Syntax of calueOf()
Parameter(s)
- data : Character Array to be converted to string
Return Value
Returns a new String object that contains data as the string representation of the passed argument
Overloaded Variants
Real world application of valueOf()
We can form a single String combining all the elements of char Array using valueOf()
Try it yourself
Use valueOf() to convert an object to its String representation. What did you observe? Find out the reason for this weird output and let us know in the comments below.
Recap
Here’s a quick summary of the Java string methods we conquered in the article.
List of other useful string methods for further learning
- contains(CharSequence s)
- isEmpty()
- join()
- repeat()
- startsWith() / endsWith()
- toLowerCase() / toUpperCase()
- indent()
Final thoughts
Congratulations!! on getting your Java strings game stronger. When dealing with strings in Java, you will undoubtedly feel more secure and at ease. But don't stop there; look at other methods and see if you can understand and implement them using the Learn By Doing process.
Now is the time to spread the word and allow your mates to catch up to you.