Java is an object oriented programming language
Object-oriented programming offers four useful design principles to depict real-world objects, or concepts in code, namely:
For example, you can think of the Linux operating system as a class from which flavours (objects) of Linux are created.
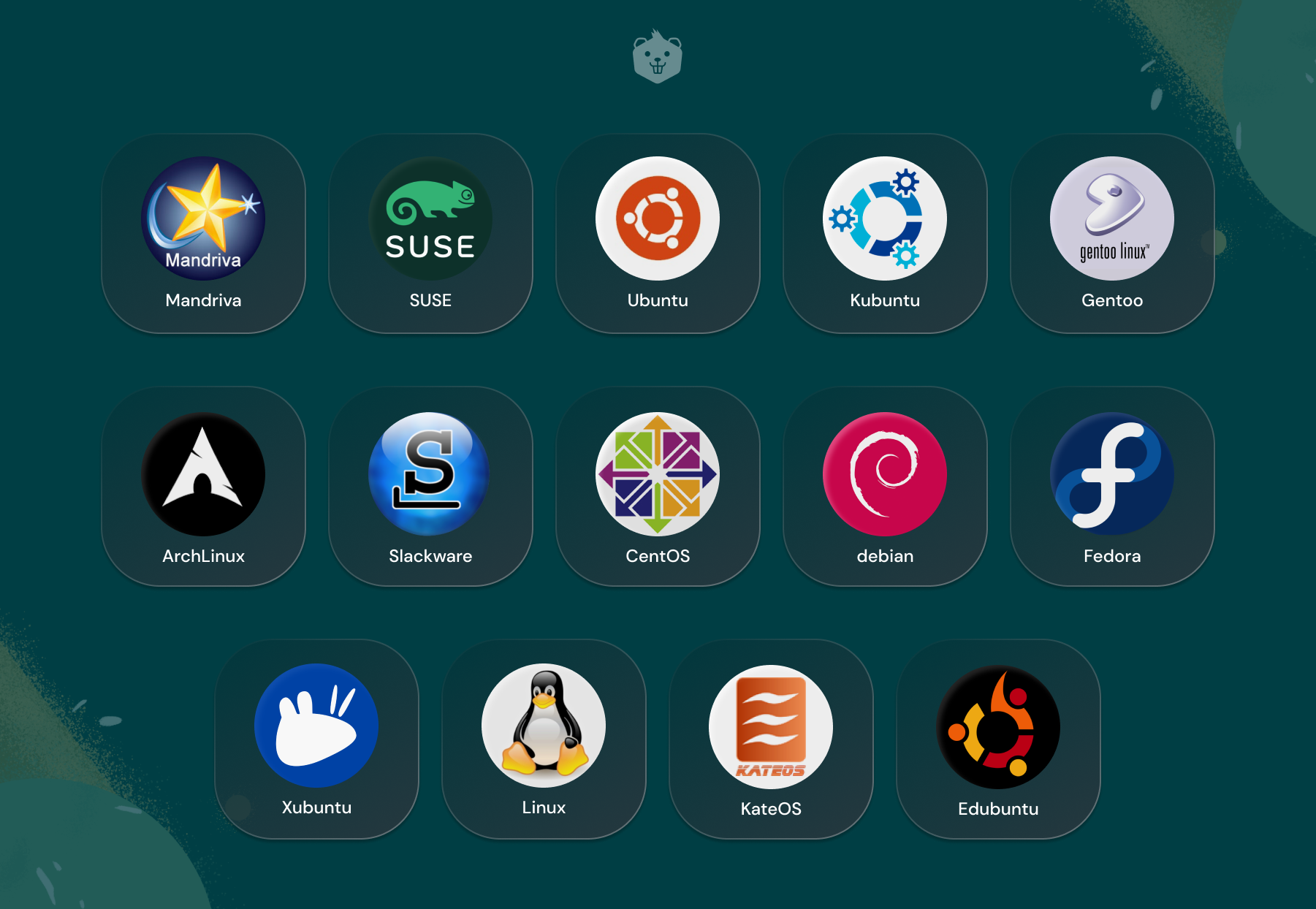
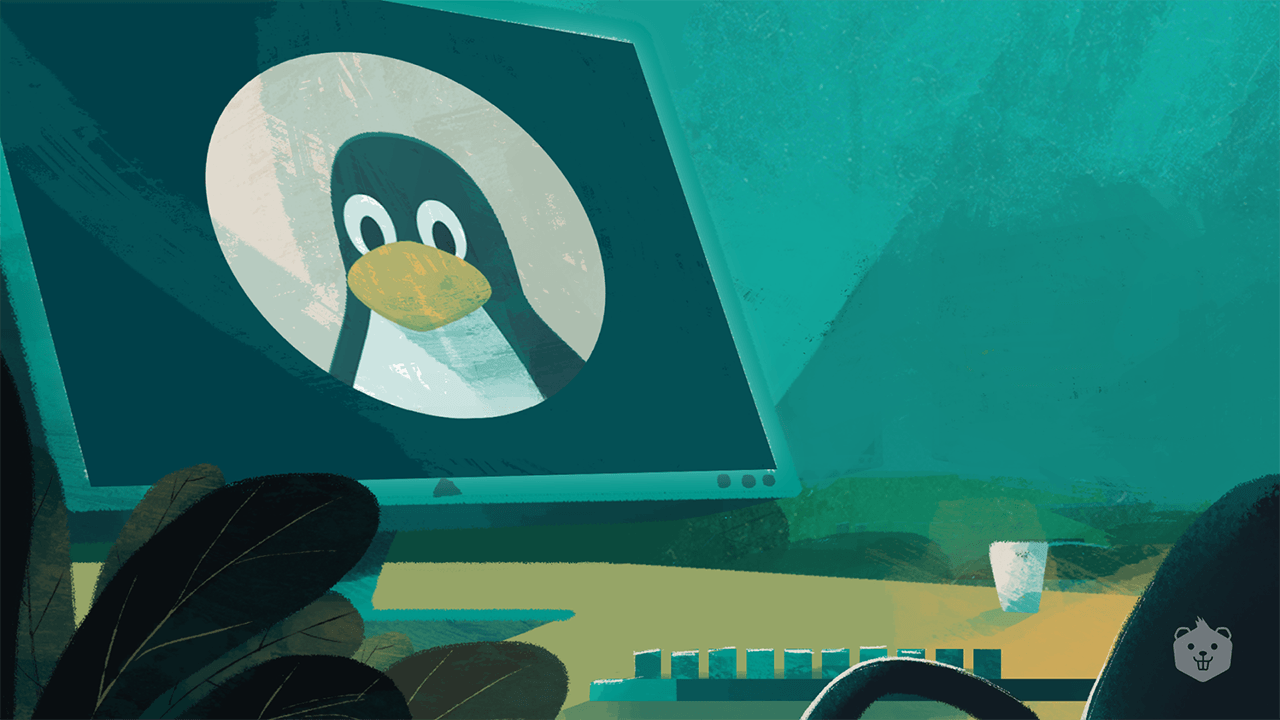
What is Abstraction?
Abstraction is a technique to identify the useful information that should be visible to a user and ignore the irrelevant details.
As a programmer, you could be designing an animation software, a car, or a heat sensor. Any such product is used by a user -- someone who cannot, and need not understand the internal working of your product.
You can set an alarm on your clock app present in your phone, within a few seconds. You need not know how the clock app works in order to use it.
Say you are looking for an application to edit pictures. A built-in app on your phone provides you with basic editing options such as filtering, enhancing, cropping, etc., But what if you’re a photographer? You might look for an application that isn't as abstract, like Adobe Photoshop, since it provides more technical features to edit photos.
There are also some apps that reduce abstraction even more, by letting the user code in their application.
Therefore, abstraction is a way humans deal with complexity.
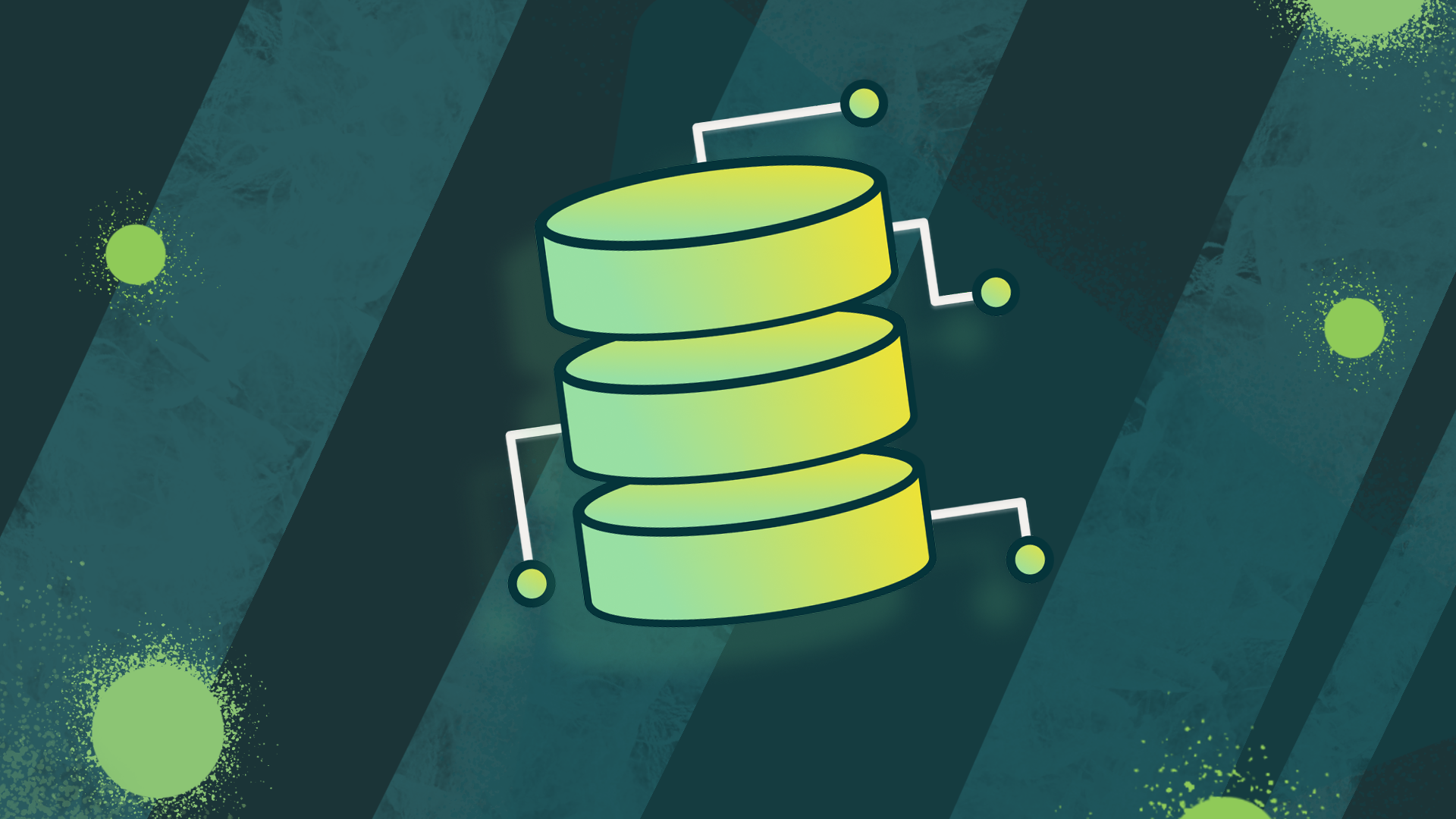
Prerequisites for Abstraction in Java
Inheritance
A subclass can inherit the properties of a superclass in Java, using the extends keyword.
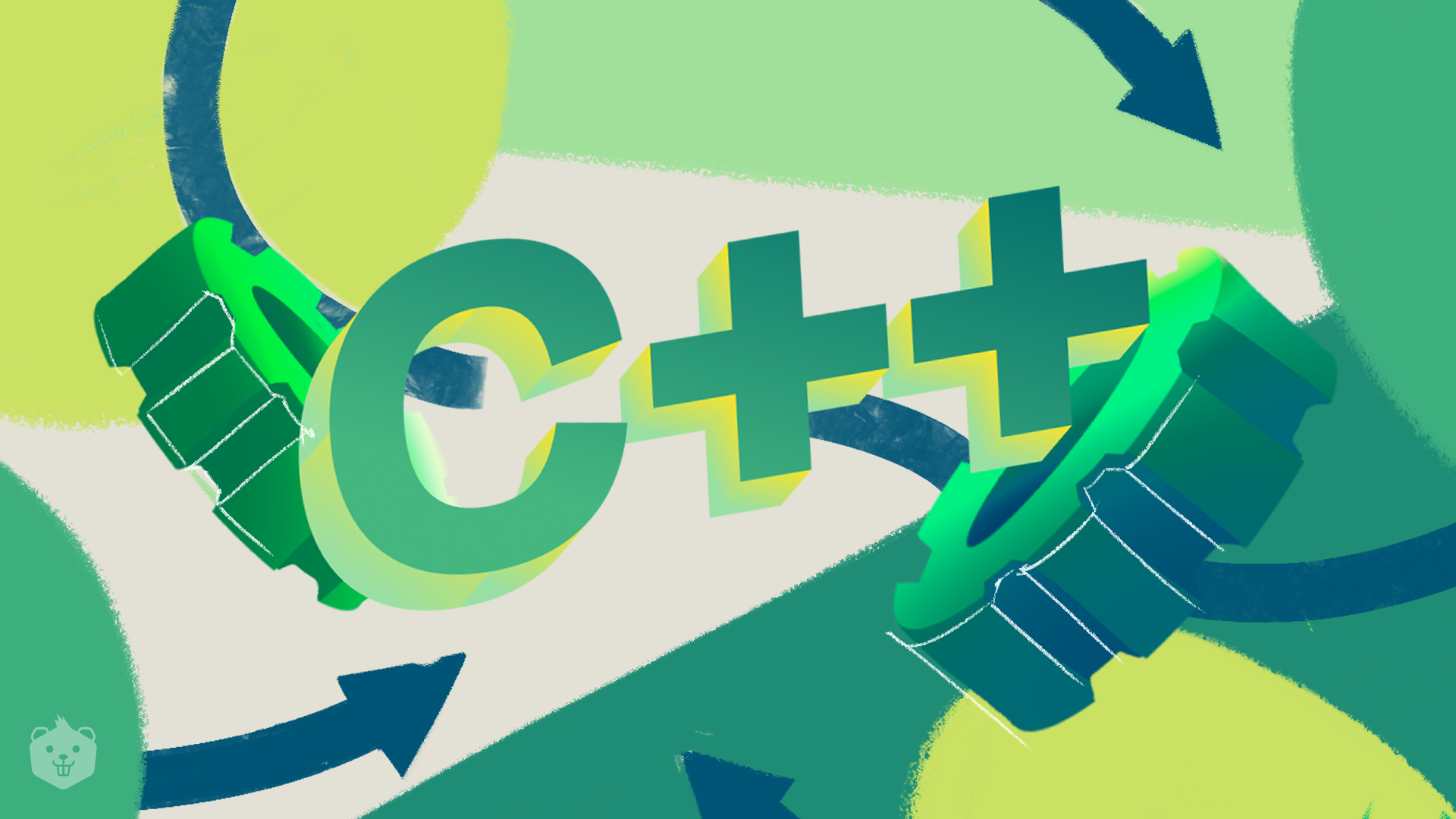
Method Overriding
A subclass that inherits the properties of a superclass can override (modify) the implementation of the methods present in the superclass
Implementing abstraction in Java
Abstraction in Java can be achieved in two ways:
1. Using abstract classes
Abstract classes achieve partial abstraction as concrete methods can also be defined in them.
2. Using interfaces
Interfaces achieve complete abstraction as only abstract methods can be defined in them.
Abstraction using Abstract Class
Abstract class
It is a class that cannot be instantiated. That is, you cannot create objects of an abstract class using the new keyword.
Abstract method
It is a method that has no code block.
You can implement abstraction in Java by declaring a class as abstract using the abstract keyword. Abstract methods are generally declared in abstract classes. But implemented methods can also be written in them. It is not compulsory to include abstract methods in abstract classes. Abstract methods (unimplemented methods) are declared using the abstract keyword.
General Syntax:
public class abstract class_name {
public abstract returnType method_name();
}
public class subclass_name extends abstract_class {
public returnType method_name() {
//method implementation
}
}
When should you use abstract classes?
Abstract classes should mainly be used when:
- You need different implementations of attribute(s) or method(s) across different subclasses that implement these attribute(s) or method(s).
- You have a design in mind, but you don’t know how to (or maybe you cannot) implement it yet.
Examples of abstraction using abstract class
Usage 1
Here, an abstract class Shape has an abstract method getArea(). The subclasses Rectangle and Square inherit the properties of Shape, override and implement the method getArea().
Thereby, a user can simply call the method getArea() on the objects of Rectangle and Square and get their area.
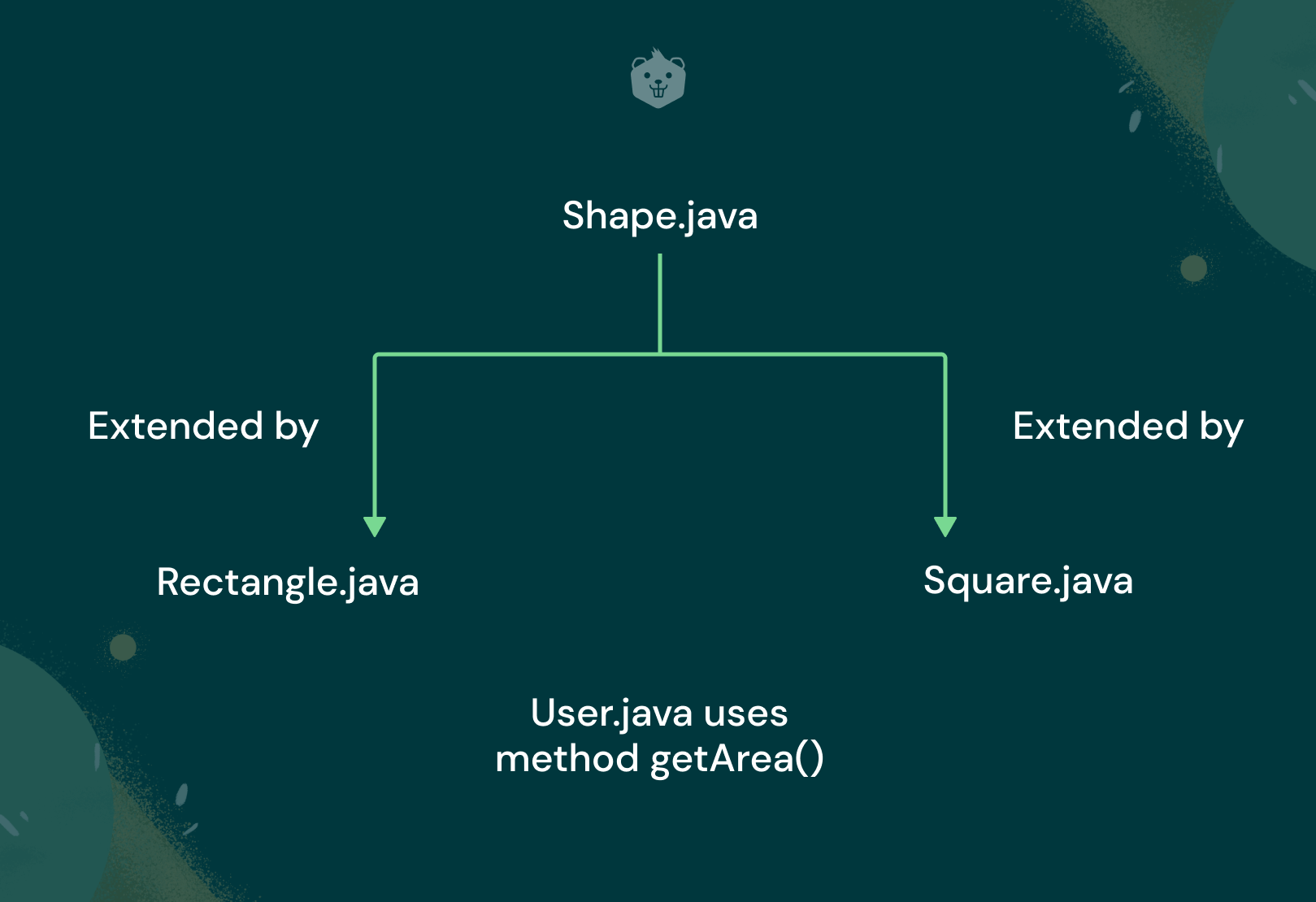
Execution steps
- Create all the classes in separate files.
- Compilation
javac class_name.java
- Running
java class_name.java
Shape.java
package pack;
public abstract class Shape {
//abstract method getArea()
public abstract double getArea();
}
Rectangle.java
import pack.Shape;
public class Rectangle extends Shape {
double length;
double width;
public Rectangle(double l, double w){
length = l;
width = w;
}
@Override
//getArea() is overridden and implemented by Rectangle
public double getArea(){
return length * width;
}
}
Square.java
import pack.Shape;
public class Square extends Shape {
double length;
public Square(double l){
length = l;
}
@Override
//getArea() is overridden and implemented by Rectangle
public double getArea(){
return length * length;
}
}
User.java
import pack.*;
public class User{
public static void main(String[] args){
Rectangle rect = new Rectangle(4, 5);
System.out.println(rect.getArea());
Square sq = new Square(3);
System.out.println(sq.getArea());
}
}
Usage 2
Think that you are designing the Instagram app, and you have an idea for a feature -- Reels. (Reels are 15-second videos that users can upload to their profile)
Reels have the potential to become popular and to be used a lot by users.
But, your company, Instagram, currently doesn't have the storage space required to be able to host millions of videos on its servers. But you need to launch Instagram to the market very soon, as the timing is perfect for it.
When the time comes, and when you have enough storage, you want to launch the Reels feature.
Java can help you here by making the method that should implement Reels as abstract. Using inheritance, you can extend the class that has the abstract Reels method, and override it and implement it in the subclass.
Thereby, you have ensured that the Reels upgrade to Instagram occurs smoothly.
Instagram.java
package pack;
public abstract class Instagram{
public String logoColor = "pink";
public String logoFont = "Roboto";
public void photoPost(){
System.out.println("Feature enabling users to post pictures");
}
public void chat(){
System.out.println("Feature enabling users to chat");
}
public void storyPost(){
System.out.println("Feature enabling users to post stories");
}
public abstract void Reels();
}
featureReels.java
import pack.Instagram;
public class featureReels extends Instagram{
public void Reels(){
System.out.println("Feature enabling users to post reels");
}
public static void main(String args[]){
featureReels reel = new featureReels();
reel.Reels();
}
}
Output
Feature enabling users to post Reels.
6 rules to follow when implementing Abstraction using abstract classes and methods
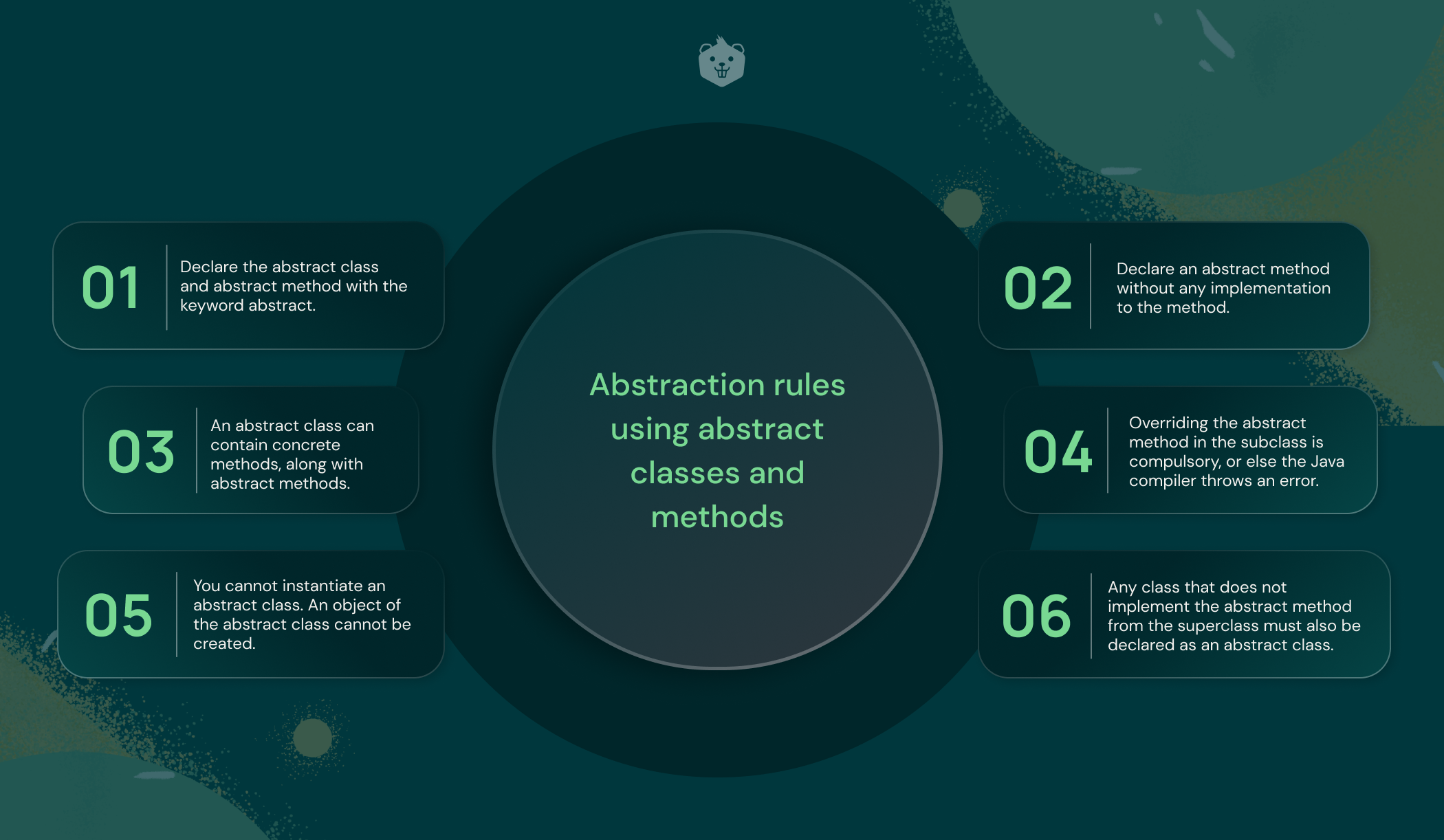
- Declare the abstract class and abstract method with the keyword abstract.
- Declare an abstract method without any implementation to the method.
- An abstract class can contain concrete methods, along with abstract methods.
- Overriding the abstract method in the subclass is compulsory, or else the Java compiler throws an error.
- You cannot instantiate an abstract class. An object of the abstract class cannot be created.
- Any class that does not implement the abstract method from the superclass must also be declared as an abstract class.
Abstraction using Interfaces
If you have followed the blog so far, understanding abstraction using interfaces is going to be very easy.
Interface
Interface is like a blueprint of a class itself. It is a mechanism to achieve abstraction. A class implements an interface.
An interface can only have abstract methods and final attributes. All the abstract methods of an interface must be implemented unless the class implementing the interface is an abstract class.
Methods that are declared in an interface are abstract by default.
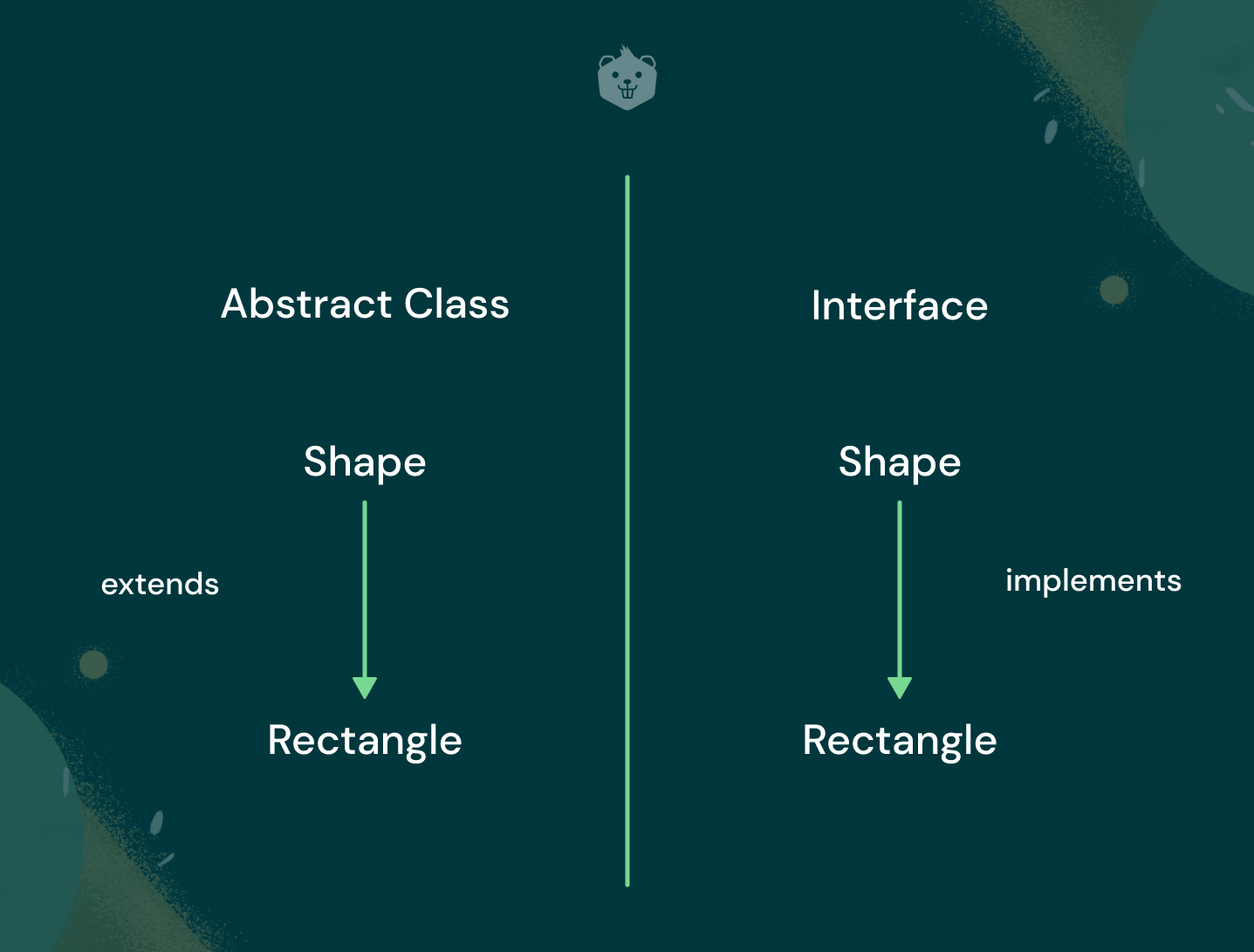
General Syntax:
public interface interface_name{
public abstract returnType method_name();
//or
public returnType method_name();
}
class class_name implements interface_name{
public returnType method_name() {
//method implementation
}
When should you use interfaces?
Interfaces are used especially when multiple inheritance is needed. Multiple inheritance occurs when a subclass inherits the properties of more than one superclass.
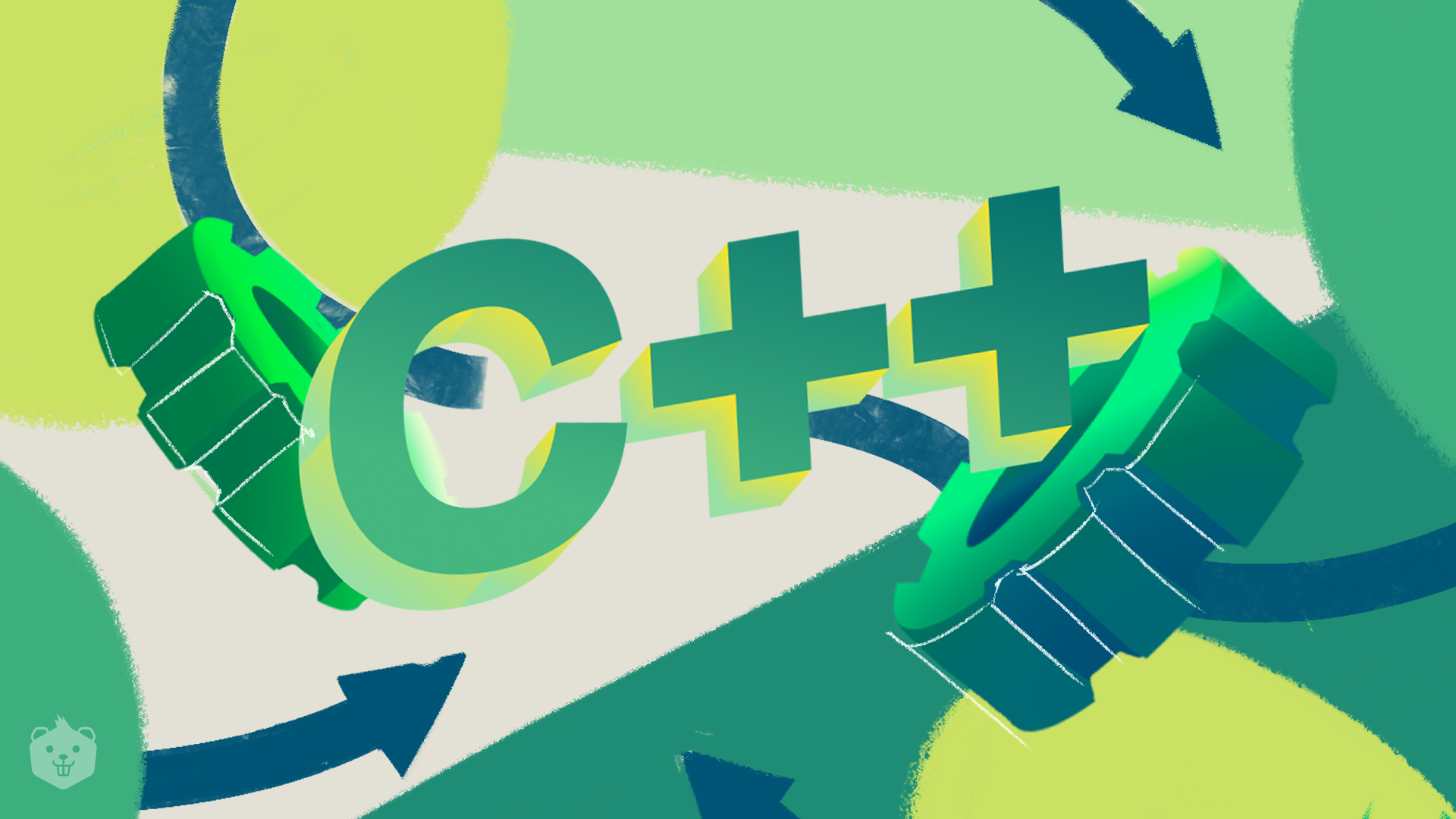
Java does not support multiple inheritance using abstract class or classes in general as the ‘diamond problem’ can occur.
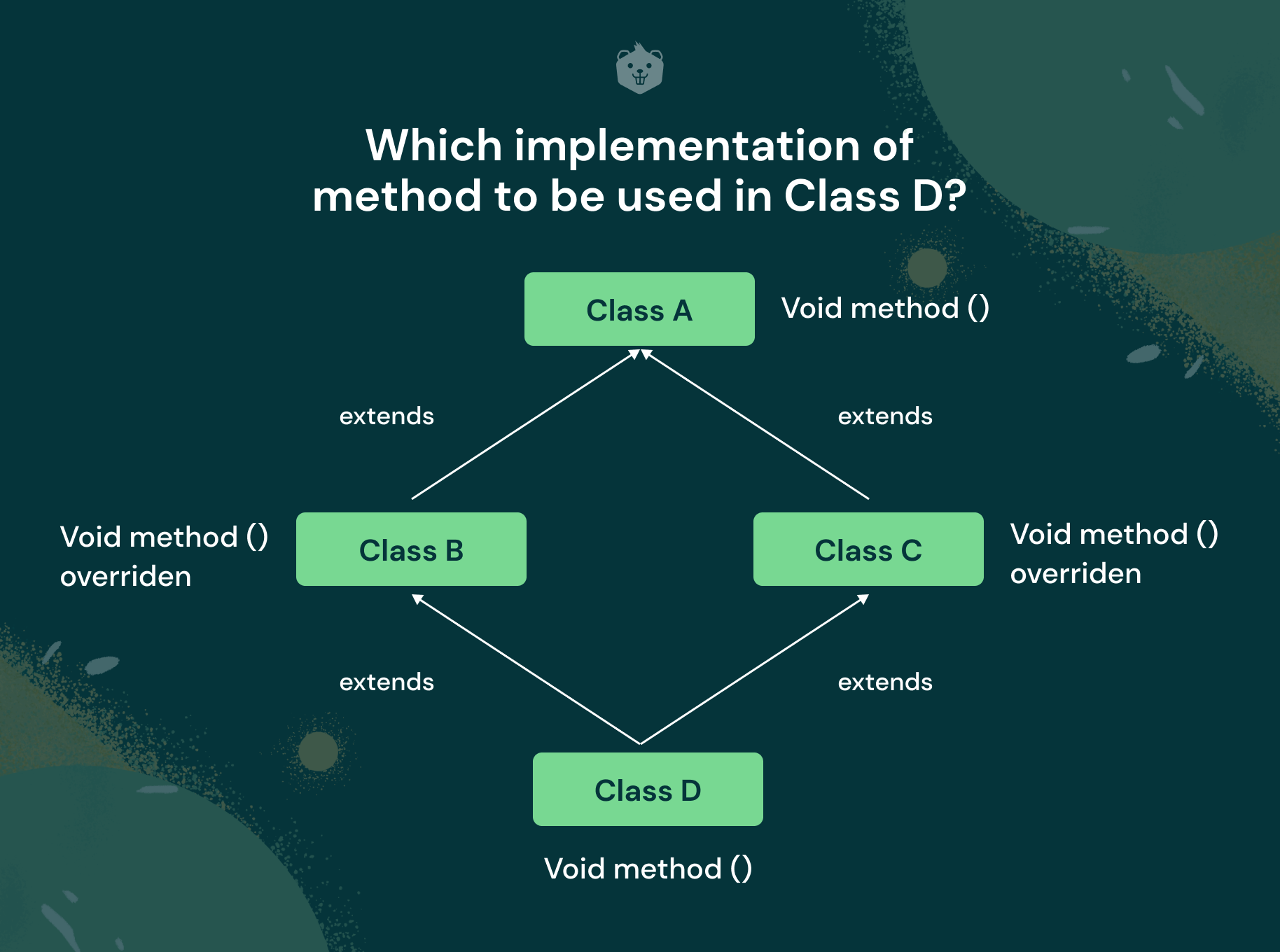
- Use interfaces if you expect unrelated classes to implement an interface.
- You want to provide a blueprint of some functions that need to be performed, but you are not concerned about who implements this blueprint.
- Interfaces are also used when you want to have multiple implementations that users can easily switch between. For example, a waiter in a restaurant can be thought of as an interface, who can take different kinds of food orders from the customers.
Examples of abstraction using interface
Here, an interface lightSwtich has two methods switchON and switchOFF, which are implemented by a class lightBulb.
A class User makes use of these methods, without knowing how the light gets turned on or off.
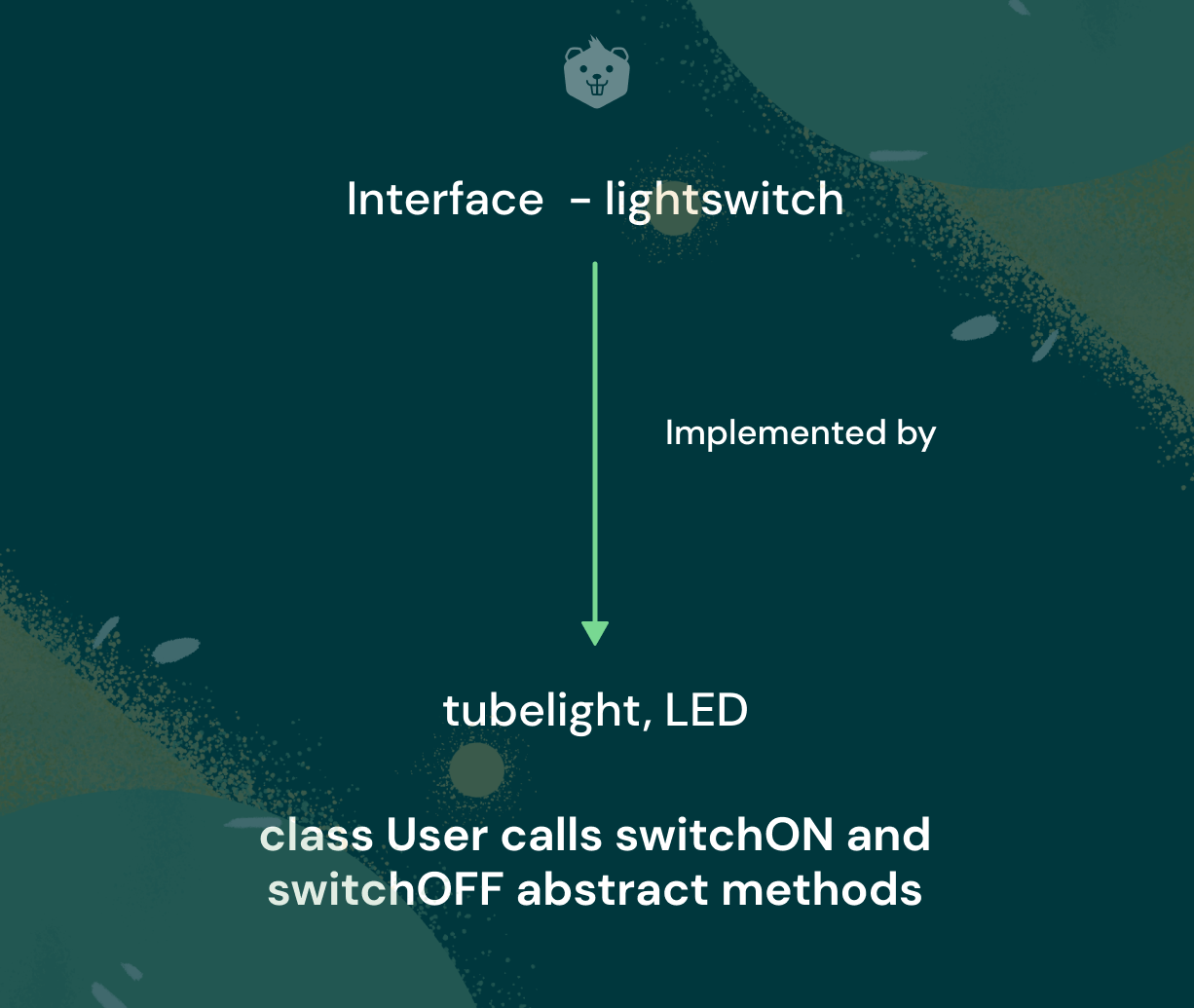
lightSwitch.java
package pack;
public interface lightSwitch {
public Boolean switchON();
public Boolean switchOFF();
}
tubeLight.java
import pack.*;
public class tubeLight implements lightSwitch {
public Boolean light;
public Boolean switchON(){
light = true;
return light;
}
public Boolean switchOFF(){
light = false;
return light;
}
}
LED.java
import pack.*;
public class LED implements lightSwitch {
public Boolean light;
public Boolean switchON(){
light = true;
return light;
}
public Boolean switchOFF(){
light = false;
return light;
}
}
User.java
import pack.*;
public class User {
public static void main (String args[]){
lightSwitch bulb1 = new tubeLight();
lightSwitch bulb2 = new LED();
System.out.println(bulb1.switchON());
System.out.println(bulb2.switchOFF());
}
}
7 rules to follow when using interfaces
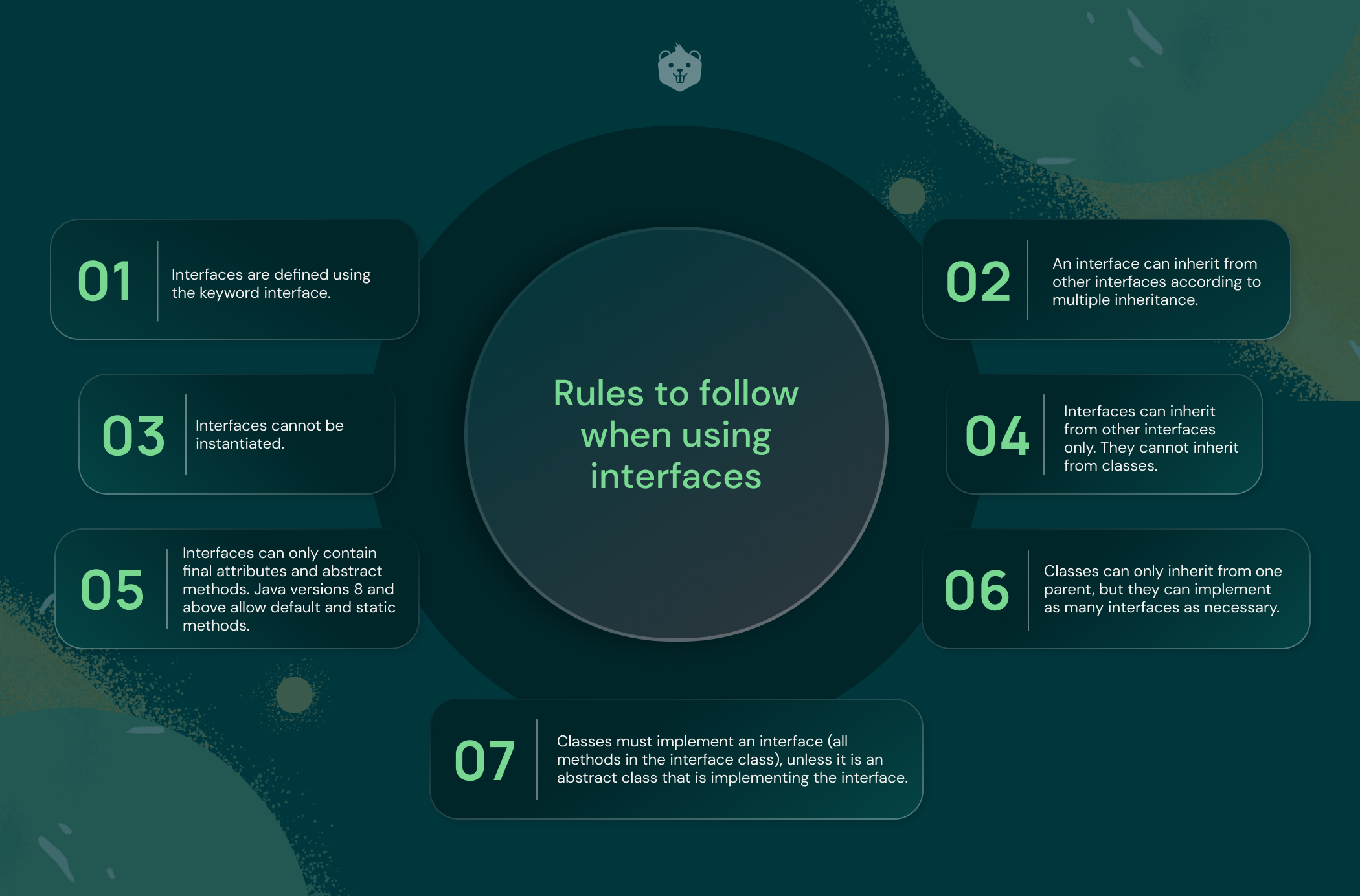
- Interfaces are defined using the keyword interface.
- Interfaces cannot be instantiated.
- Interfaces can only contain final attributes and abstract methods. Java versions 8 and above allow default and static methods.
- Classes must implement an interface (all methods in the interface class), unless it is an abstract class that is implementing the interface.
- Classes can only inherit from one parent, but they can implement as many interfaces as necessary.
- Interfaces can inherit from other interfaces only. They cannot inherit from classes.
- An interface can inherit from other interfaces according to multiple inheritance.
Test Your Understanding
Q1.Abstraction works more on the design level than the implementation level in software design
A.True
B.False
Q2. Apply abstraction to the method “explore” in the code snippets given below by using the concept of abstract classes.
CrioBlog.java
package pack;
public class CrioBlog{
public String url = "https://www.crio.do/blog/";
public void explore(){
System.out.println(“Crio Blogs help you to read about a topic and master it ! Learn By Doing!”);
}
}
MiniProjects.java
import pack.CrioBlog
public class MiniProjects extends CrioBlog{
public String url = "https://www.crio.do/blog/tag/mini-projects-for-cse/";
public void explore(){
System.out.println("List of free and unique mini project topics for beginners and advanced developers. Project ideas in Python, Java, Web Development, Machine Learning, more");
}
}
Main.java
import pack.*
public class Main {
public static void main(String args[]){
CrioBlog baseclass = new CrioBlog();
baseclass.explore();
MiniProjects mini = new MiniProjects();
mini.explore();
System.out.println(mini.url);
}
}
Solution :
CrioBlog.java
package pack;
public abstract class CrioBlog{
public String url = "https://www.crio.do/blog/";
public abstract void explore()
}
Main.java
import pack.*
public class Main {
public static void main(String args[]){
MiniProjects mini = new MiniProjects();
mini.explore();
System.out.println(mini.url);
}
}
Abstract class vs Interface
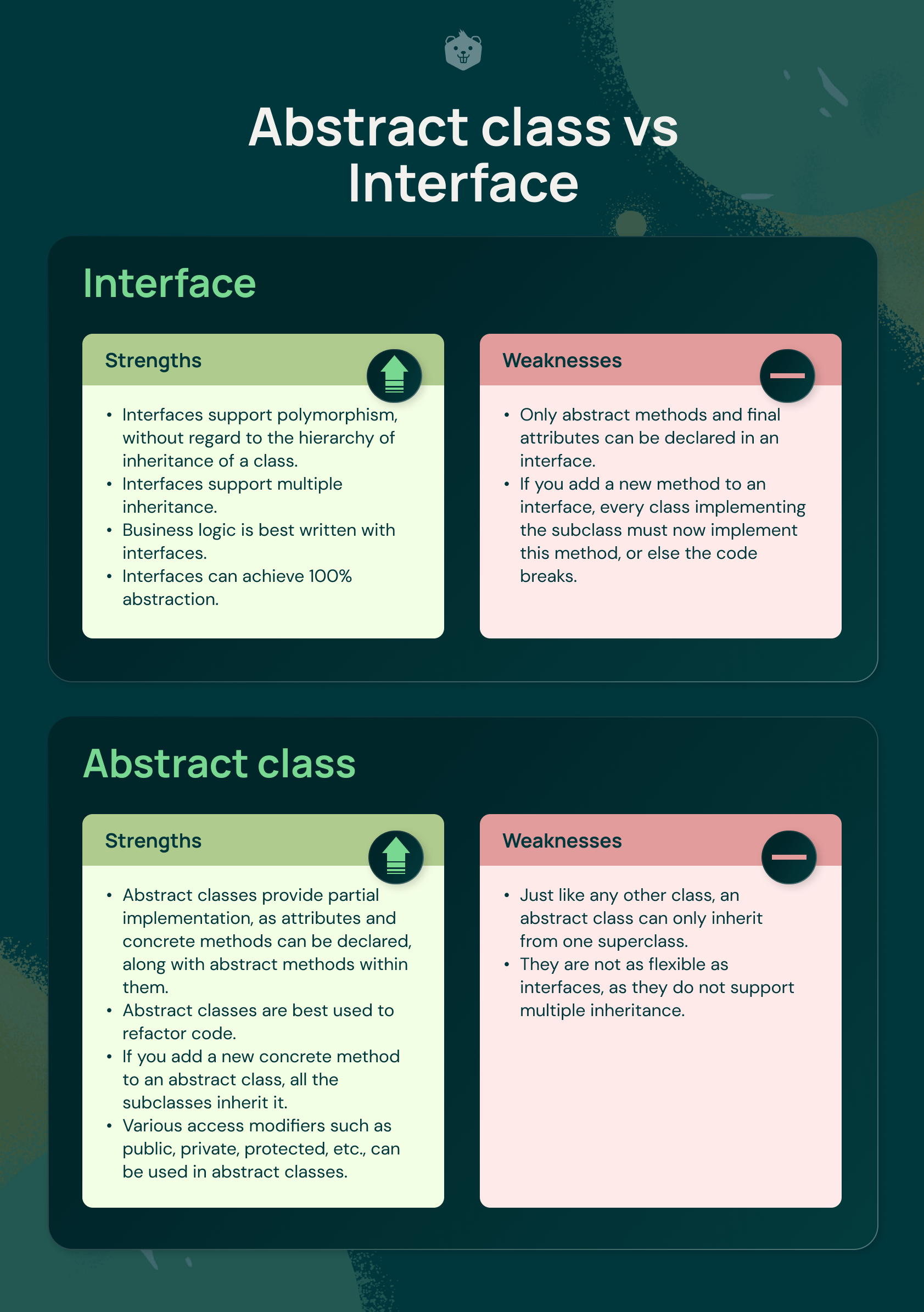
Interface
Strengths
- Interfaces support polymorphism, without regard to the hierarchy of inheritance of a class.
- Interfaces support multiple inheritance.
- Business logic is best written with interfaces.
- Interfaces can achieve 100 % abstraction.
Weaknesses
- Only abstract methods and final attributes can be declared in an interface.
- If you add a new method to an interface, every class implementing the subclass must now implement this method, or else the code breaks.
Abstract class
Strengths
- Abstract classes provide partial implementation, as attributes and concrete methods can be declared, along with abstract methods within them.
- Abstract classes are best used to refactor code.
- If you add a new concrete method to an abstract class, all the subclasses inherit it.
- Various access modifiers such as public, private, protected, etc., can be used in abstract classes.
Weaknesses
- Just like any other class, an abstract class can only inherit from one superclass.
- They are not as flexible as interfaces, as they do not support multiple inheritance.
Abstraction vs Encapsulation vs Data Hiding
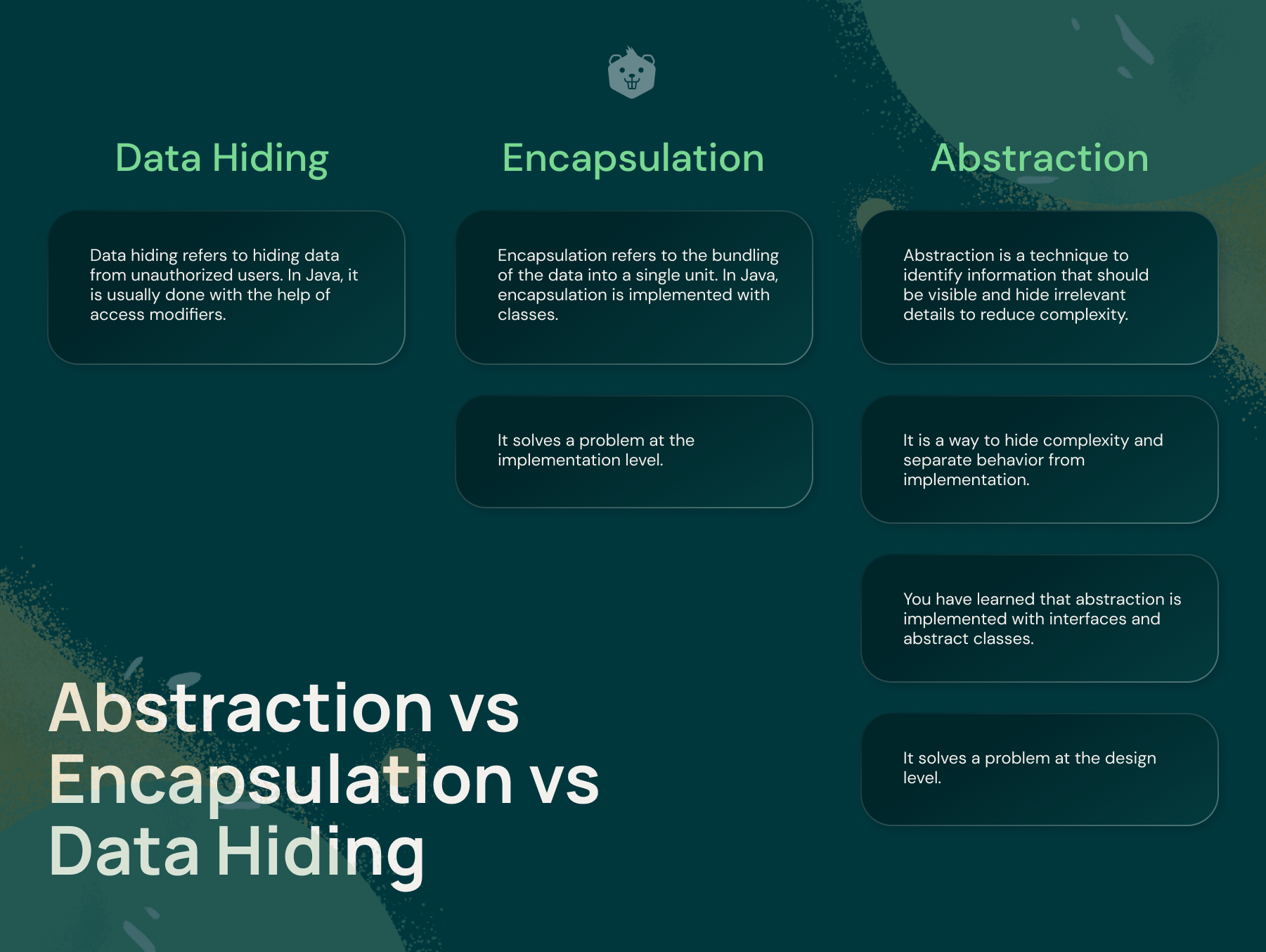
Data Hiding
Data hiding refers to hiding data from unauthorized users. In Java it is usually done with the help of access modifiers.
Encapsulation
- Encapsulation refers to the bundling of the data into a single unit. In Java, encapsulation is implemented with classes.
- It solves a problem at the implementation level.
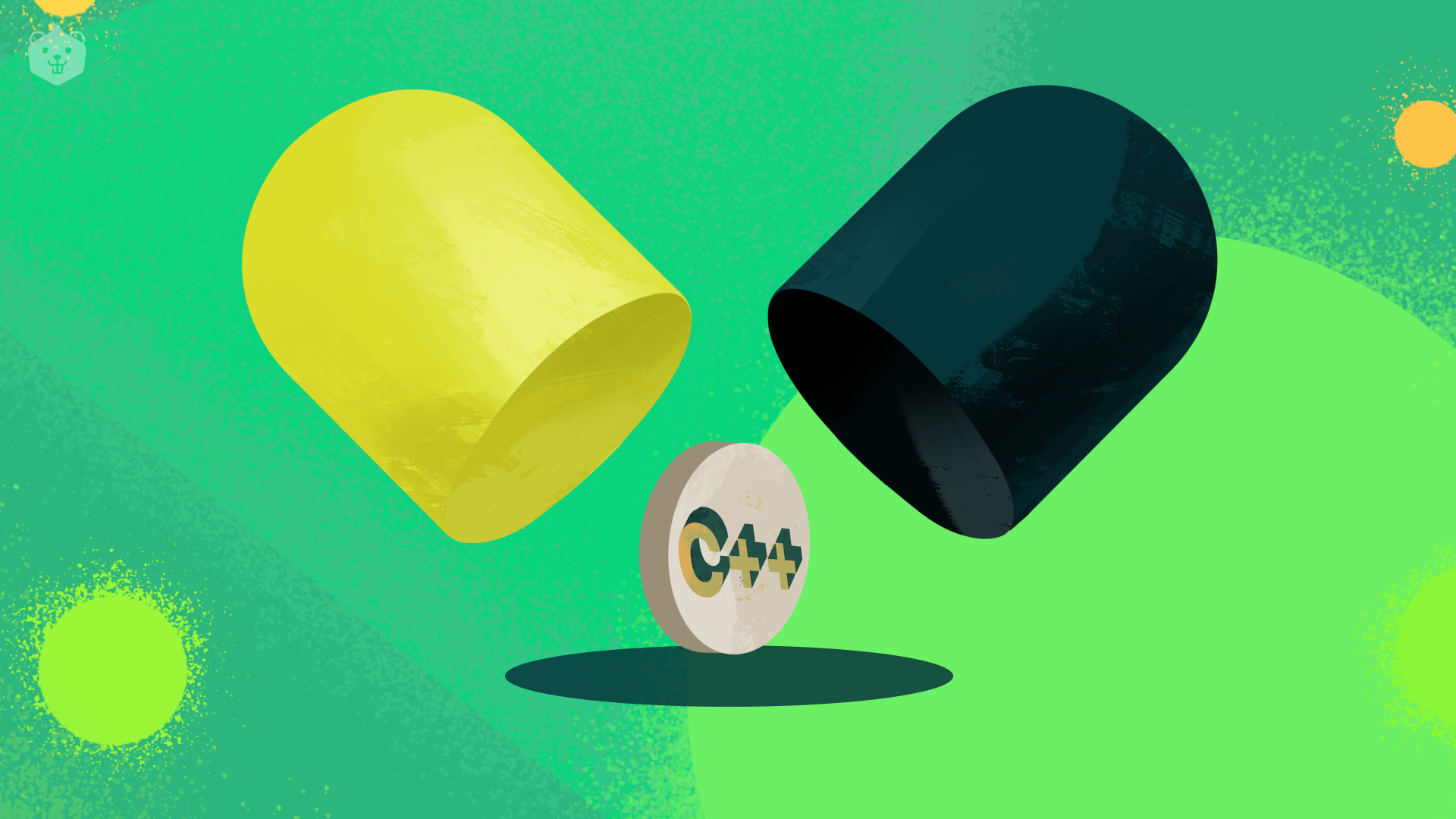
Abstraction
- Abstraction is a technique to identify information that should be visible and hide irrelevant details to reduce complexity.
- It is a way to hide complexity and separate behavior from implementation.
- You have learned that abstraction is implemented with interfaces and abstract classes.
- It solves a problem at the design level.
Test Your Understanding
Q3. Does the code snippet support multiple inheritance?
Interface A {
//code block
}
Interface B{
//code block
}
class C implements A,B{
//code block
}
Q4. Abstract classes can achieve 0-100% abstraction, while interfaces achieve 100% abstraction.
- True
- False
Q5. An abstract class can implement an interface
- True
- False
Q6. What caused this compile-time error?
“Illegal modifier for the interface field; only public, static & final are permitted”
Real-world applications of Abstraction
- Every application on your phone has user interfaces that are designed keeping abstraction in mind. A user basically sets off a complex operation at the tap of a button on a screen. This complexity is hidden from the user and only relevant information is displayed.
- Abstraction often uses the idea of dividing an entity into components. For example, abstraction for a mobile phone would include dividing it into components such as touch-screen, Wifi, audio-jack, etc., Related components are chosen and encapsulated into classes so that they interact with each other through a fixed interface
- An ATM machine is a great example of abstraction. A user can withdraw money from the machine, by interacting with only relevant information such as entering an ATM pin, without having to know the internal mechanism of the ATM.
- Using the internet itself is a form of abstraction. We are not even consciously thinking about the massive optical fibers running across miles under the sea, moving internet traffic across the world.
Do it Yourself
- Design a simple application that needs to extend an abstract class, as well as implement an interface.
- Consider a scenario of a customer and a shopkeeper. Describe abstraction in this relationship in terms of an interface for a customer object and a shopkeeper object.
Further Learning
Learn how Abstraction is used in real-world scenarios with a series of fun activities
Start Now, it's free!Related reads
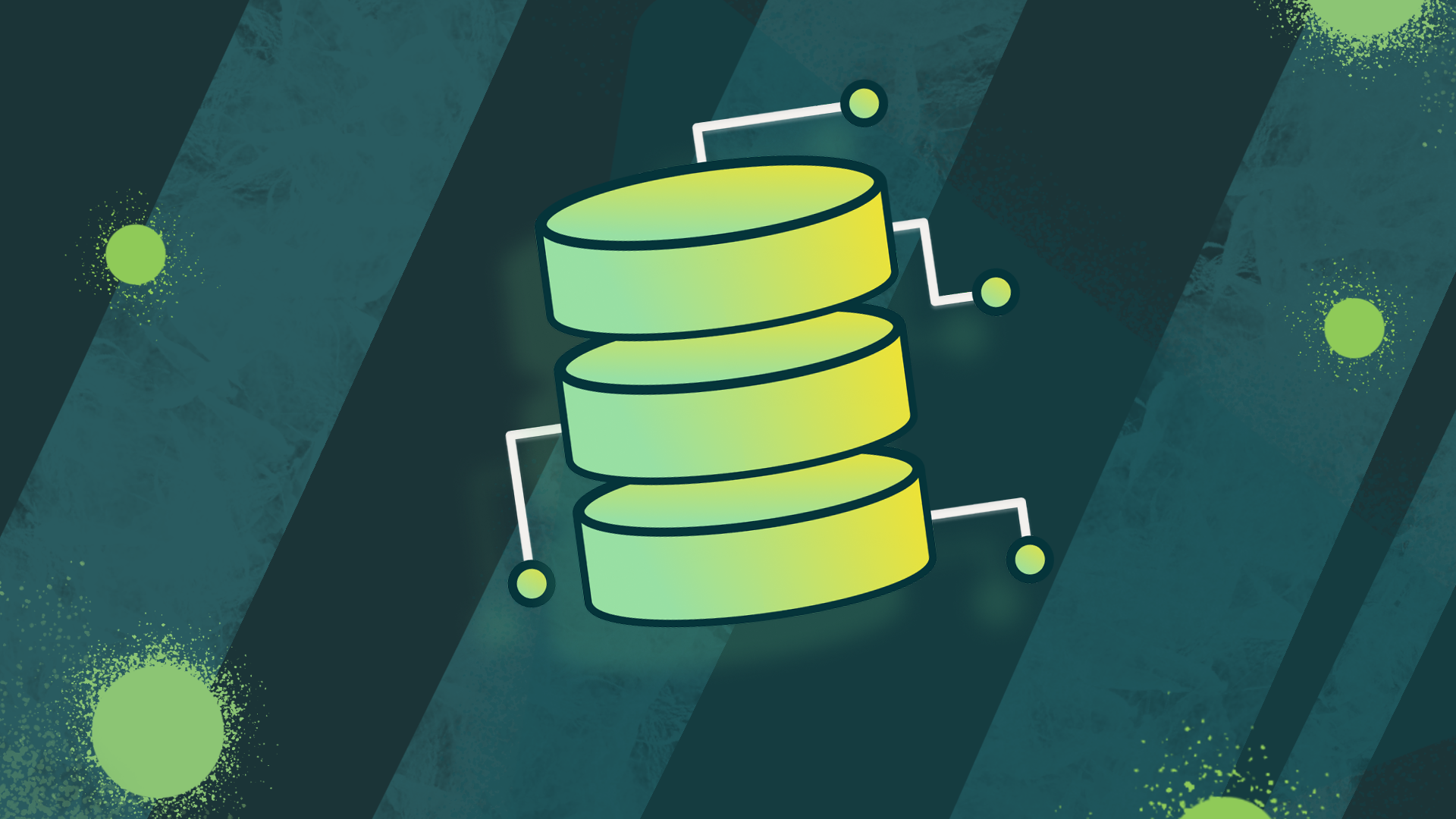
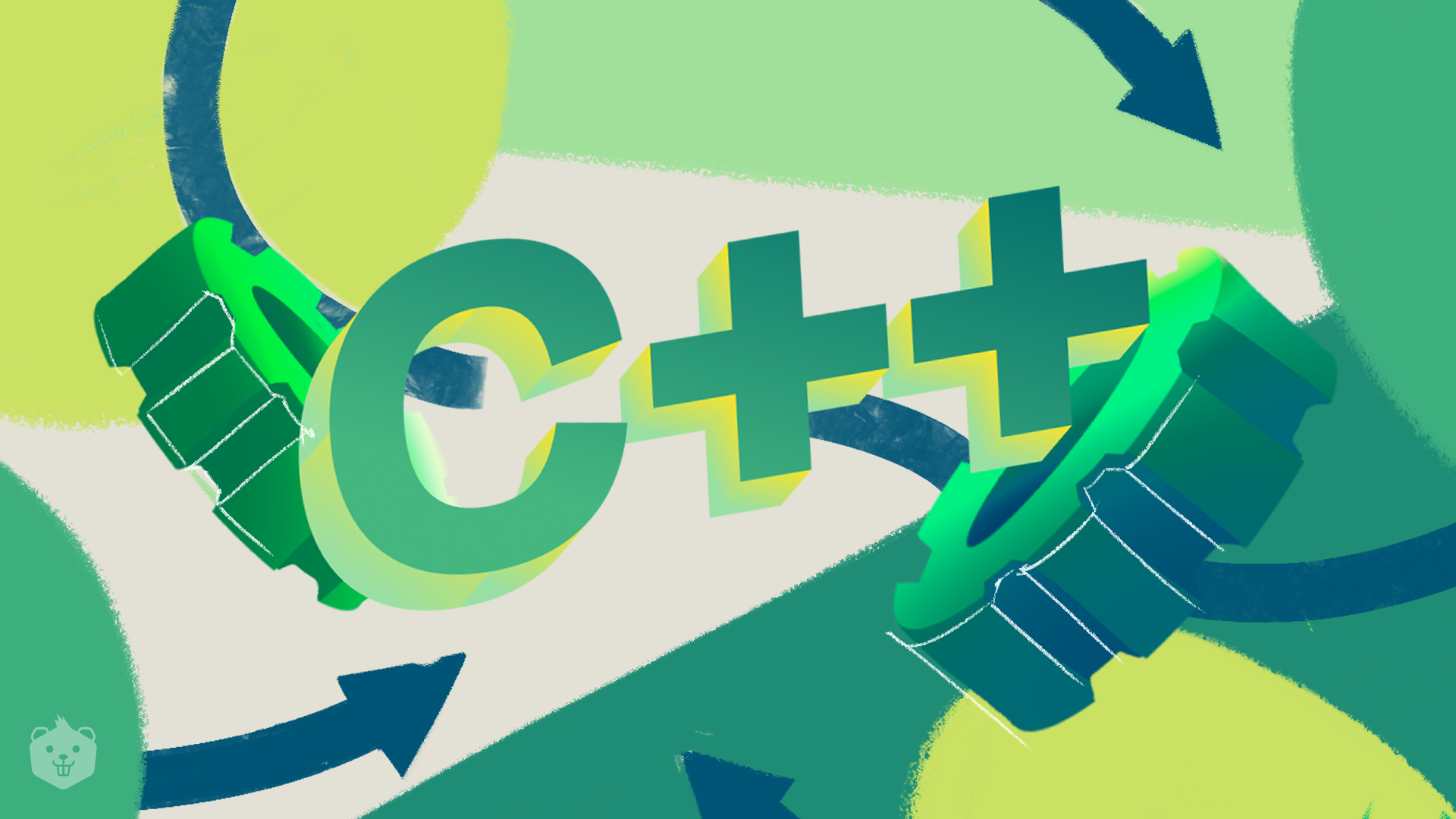
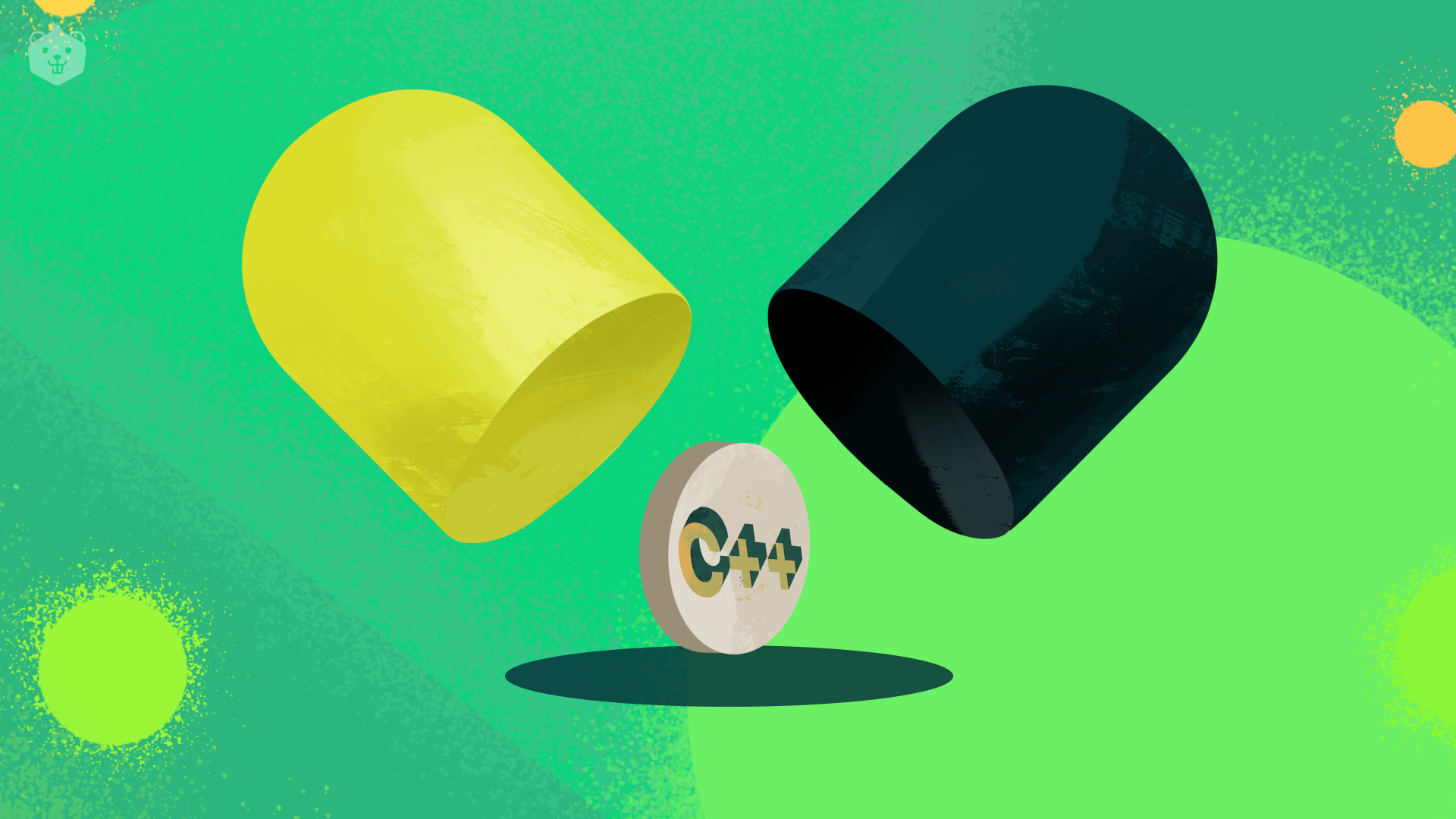
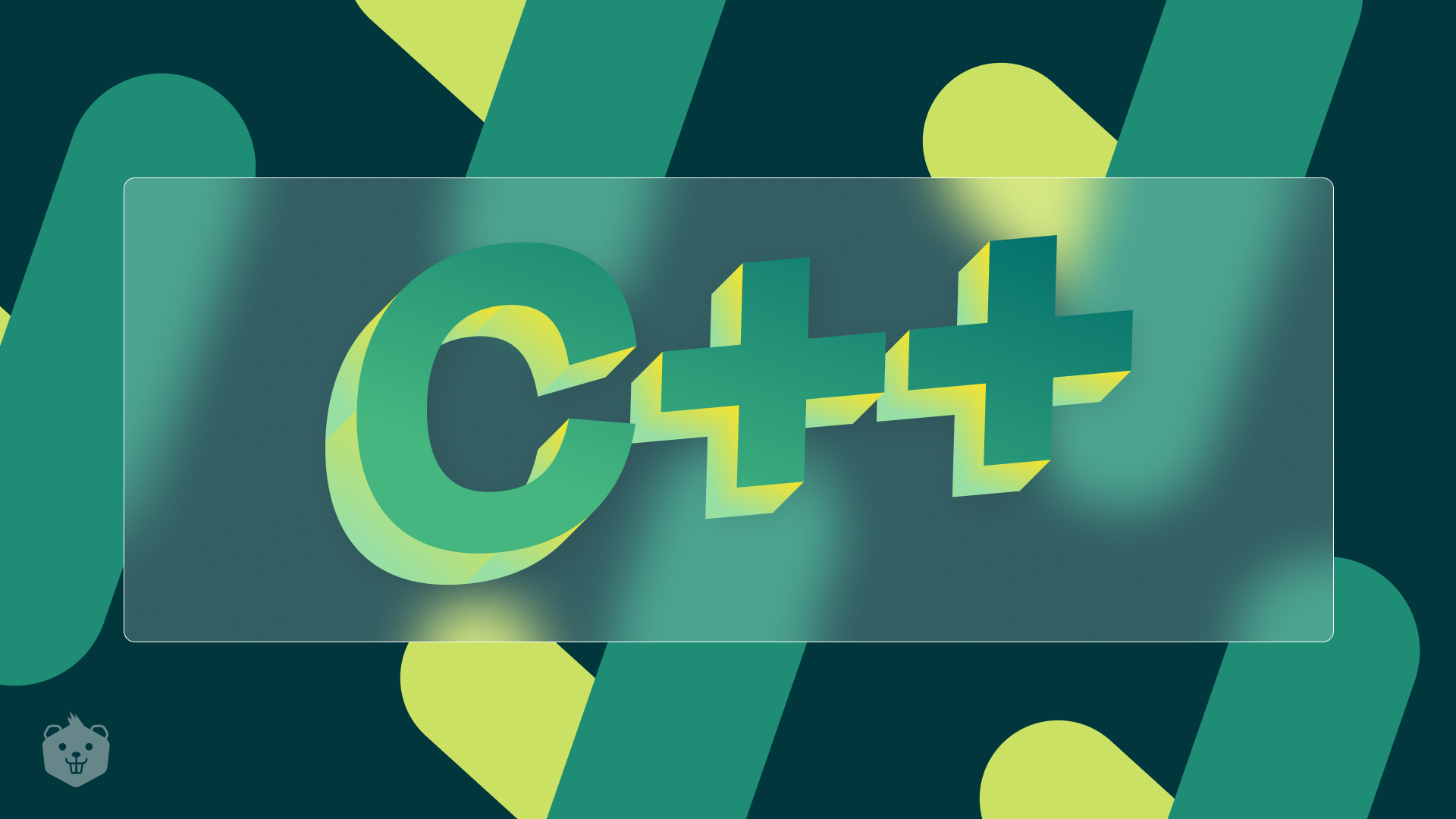